用c语言写一个贪吃蛇游戏
时间: 2024-05-07 11:17:17 浏览: 10
好的,我可以为您提供一个基本的贪吃蛇游戏的 C 语言代码。以下是代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
// 定义游戏面板的大小
const int WIDTH = 40;
const int HEIGHT = 20;
// 定义贪吃蛇的结构体
struct Snake {
int x, y; // 蛇头的坐标
struct Snake *next; // 指向下一个蛇节的指针
};
// 定义各种游戏的全局变量
int score = 0; // 记分
int speed = 200; // 游戏速度
char direction = 'd'; // 蛇的前进方向
struct Snake *head, *tail; // 蛇头与蛇尾的指针
int food_x, food_y; // 食物的坐标
// 生成随机数
int rand_int(int min, int max) {
return rand() % (max - min + 1) + min;
}
// 在指定位置输出字符
void gotoxy(int x, int y) {
COORD coord;
coord.X = x;
coord.Y = y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), coord);
}
// 初始化游戏
void game_init() {
// 隐藏光标
CONSOLE_CURSOR_INFO cursor_info = {1, 0};
SetConsoleCursorInfo(GetStdHandle(STD_OUTPUT_HANDLE), &cursor_info);
// 初始化蛇
head = (struct Snake *)malloc(sizeof(struct Snake));
head->x = WIDTH / 2;
head->y = HEIGHT / 2;
head->next = NULL;
tail = head;
// 随机生成食物
food_x = rand_int(1, WIDTH - 2);
food_y = rand_int(1, HEIGHT - 2);
}
// 画出游戏面板
void draw_panel() {
// 画出四条边框线
for (int i = 0; i < HEIGHT; i++) {
gotoxy(0, i);
printf("#");
gotoxy(WIDTH - 1, i);
printf("#");
}
for (int i = 1; i < WIDTH - 1; i++) {
gotoxy(i, 0);
printf("#");
gotoxy(i, HEIGHT - 1);
printf("#");
}
// 画出游戏信息
gotoxy(WIDTH + 5, 5);
printf("Score: %d", score);
gotoxy(WIDTH + 5, 7);
printf("Speed: %d ms", speed);
}
// 画出蛇和食物
void draw_snake_and_food() {
// 清空原来的蛇和食物
struct Snake *p = tail;
while (p != head) {
gotoxy(p->x, p->y);
printf("*");
p = p->next;
}
gotoxy(head->x, head->y);
printf("@");
gotoxy(food_x, food_y);
printf("O");
}
// 在指定位置输出字符串
void print_text(int x, int y, char *text) {
gotoxy(x, y);
printf("%s", text);
}
// 检查游戏是否结束
bool check_gameover() {
// 撞墙了
if (head->x <= 0 || head->x >= WIDTH - 1 || head->y <= 0 || head->y >= HEIGHT - 1) {
print_text(WIDTH + 5, 10, "Game Over!");
return true;
}
// 撞到自己了
struct Snake *p = tail;
while (p != head) {
if (p->x == head->x && p->y == head->y) {
print_text(WIDTH + 5, 10, "Game Over!");
return true;
}
p = p->next;
}
return false;
}
// 游戏主循环
void game_loop() {
while (1) {
// 检查是否得分
if (head->x == food_x && head->y == food_y) {
score++;
// 新增一个蛇节
struct Snake *node = (struct Snake *)malloc(sizeof(struct Snake));
node->x = tail->x;
node->y = tail->y;
node->next = NULL;
tail->next = node;
tail = node;
// 随机生成新的食物
food_x = rand_int(1, WIDTH - 2);
food_y = rand_int(1, HEIGHT - 2);
// 加速
speed -= 10;
if (speed <= 50) speed = 50;
}
// 检查游戏是否结束
if (check_gameover()) {
break;
}
// 根据方向移动蛇
switch (direction) {
case 'w':
head->y--;
break;
case 'a':
head->x--;
break;
case 's':
head->y++;
break;
case 'd':
head->x++;
break;
}
// 清空原来的蛇和食物
draw_snake_and_food();
// 延时一段时间
Sleep(speed);
// 判断用户输入的方向
if (kbhit()) {
char ch = getch();
if ((ch == 'w' && direction != 's') ||
(ch == 'a' && direction != 'd') ||
(ch == 's' && direction != 'w') ||
(ch == 'd' && direction != 'a')) {
direction = ch;
}
}
}
}
// 释放内存
void game_over() {
// 释放蛇节点的内存
struct Snake *p = head;
while (p != NULL) {
tail = p->next;
free(p);
p = tail;
}
// 显示光标
CONSOLE_CURSOR_INFO cursor_info = {1, 1};
SetConsoleCursorInfo(GetStdHandle(STD_OUTPUT_HANDLE), &cursor_info);
}
int main() {
game_init();
draw_panel();
draw_snake_and_food();
game_loop();
game_over();
return 0;
}
```
以上是一个简单的 C 语言贪吃蛇游戏代码,您可以根据自己的需求对它进行修改和扩展。
相关推荐
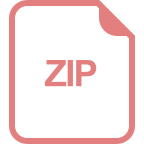
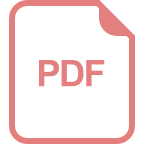
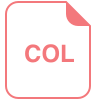
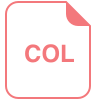
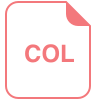
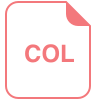
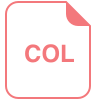


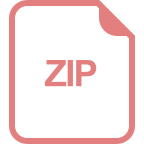
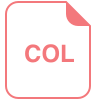
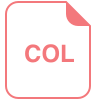
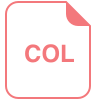
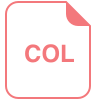
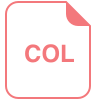