int SetUpTCPtoSendInformation(char option[], char *command) { static char *TCPCommand; if (strstr(option, "set")) { TCPCommand = malloc(strlen(command) + 1); if (TCPCommand == NULL) { CON_LOG("Failed to set and obtain TCP command variable memory allocation"); return 0; } strcpy(TCPCommand, command); return 1; } else if (strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand)) { strcpy(command, TCPCommand); memset(TCPCommand, '\0', strlen(TCPCommand)); free(TCPCommand); return 1; } memset(command,0,sizeof(command)); strcpy(command, ""); return 0; } 上面使用锁避免多个进程同时访问
时间: 2024-04-08 20:34:03 浏览: 21
在上面的代码中,并没有使用锁来避免多个进程同时访问。你可以使用互斥锁(mutex)来实现对共享资源的同步访问。下面是一个使用互斥锁的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <pthread.h>
static char *TCPCommand;
static pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
int SetUpTCPtoSendInformation(char option[], char *command) {
pthread_mutex_lock(&mutex); // 加锁
if (strstr(option, "set")) {
TCPCommand = malloc(strlen(command) + 1);
if (TCPCommand == NULL) {
CON_LOG("Failed to set and obtain TCP command variable memory allocation");
pthread_mutex_unlock(&mutex); // 解锁
return 0;
}
strcpy(TCPCommand, command);
pthread_mutex_unlock(&mutex); // 解锁
return 1;
} else if (strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand)) {
strcpy(command, TCPCommand);
memset(TCPCommand, '\0', strlen(TCPCommand));
free(TCPCommand);
pthread_mutex_unlock(&mutex); // 解锁
return 1;
}
memset(command, 0, sizeof(command));
strcpy(command, "");
pthread_mutex_unlock(&mutex); // 解锁
return 0;
}
```
在代码中,我们使用了 `pthread_mutex_t` 类型的互斥锁,并使用 `pthread_mutex_lock` 和 `pthread_mutex_unlock` 函数来分别加锁和解锁。这样可以确保在访问共享资源(`TCPCommand`)时只有一个线程可以进行操作,避免了多个进程同时访问的问题。
相关推荐
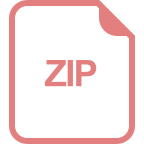
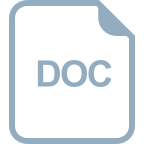
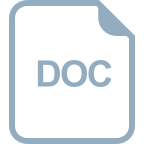









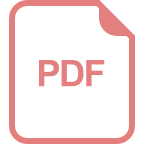
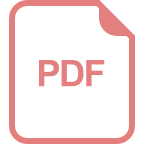
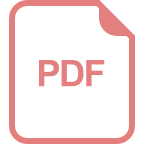
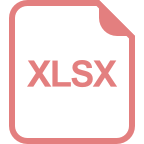
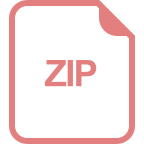