def load_pre_trained(): # load pre-trained embedding model embeddings_index = {} with open('D:\Desktop\深度学习\Embedding\sgns.sogou.word',encoding='utf-8') as f: _, embedding_dim = f.readline().split() for line in f: values = line.split() word = values[0] coefs = np.asarray(values[1:], dtype='float32') embeddings_index[word] = coefs print('Found %s 单词数量, 向量的维度信息 %s' % (len(embeddings_index), embedding_dim)) return embeddings_index
时间: 2024-04-02 16:32:15 浏览: 89
这段代码是用于加载预训练的词向量模型,其中使用的是搜狗新闻词向量数据集sgns.sogou.word。该数据集是一个预训练的中文词向量模型,包含了超过1.8亿个中文词汇及其对应的向量表示。代码中使用的是Python中的字典数据结构(embeddings_index),将每个单词和其对应的词向量存储在该字典中。最后,该函数返回了加载后的词向量模型。
相关问题
怎么理解这段代码: def load_embedding(filename): geneList = list() vectorList = list() f = open(filename) for line in f: values = line.split() gene = values[0] vector = np.asarray(values[1:], dtype="float32") geneList.append(gene) vectorList.append(vector) f.close() return np.asarray(vectorList), np.asarray(geneList) embeddings_file = "../pre_trained_emb/gene2vec_dim_200_iter_9.txt" wv, vocabulary = load_embedding(embeddings_file) indexes = list(range(len(wv))) random.shuffle(indexes) topN = len(wv) rdWV = wv[indexes][:topN,:] rdVB = vocabulary[indexes][:topN] print("PCA!") pca = PCA(n_components=50) pca.fit(rdWV) pca_rdWV=pca.transform(rdWV) print("PCA done!") print("tsne!") with open("../TSNE_label_gene2vec.txt", 'w') as out: for str in rdVB: out.write(str + "\n") out.close() def TSNEWoker (iter): print(iter+" begin") tsne = TSNE(n_components=2, perplexity=30, n_iter=int(iter), learning_rate=200, n_jobs=32) np.set_printoptions(suppress=True) # save tsne data Y = tsne.fit_transform(pca_rdWV) np.savetxt("../TSNE_data_gene2vec.txt_"+iter+".txt",Y) print(iter+" tsne done!") def mp_handler(): p = Pool(6) p.map(TSNEWoker, ["100","5000","10000","20000","50000","100000"]) #generate tsne of different iteration in parallel if __name__ == '__main__': # TSNEWoker("5000") mp_handler()
这段代码主要是用于加载嵌入矩阵数据,进行PCA降维和TSNE可视化操作。下面是代码的解释:
1. `load_embedding` 函数用于加载文件中的嵌入矩阵数据。它首先创建两个空列表 `geneList` 和 `vectorList`,然后打开指定的文件。对于文件中的每一行,它使用 `split()` 方法将行拆分为单词列表 `values`,其中第一个值是基因名称 `gene`,其余值是嵌入向量 `vector`。然后将 `gene` 添加到 `geneList` 中,将 `vector` 添加到 `vectorList` 中。最后将两个列表转换为NumPy数组并返回。
2. `embeddings_file` 是嵌入文件的路径,通过调用 `load_embedding(embeddings_file)` 加载嵌入矩阵数据,并将返回的嵌入矩阵存储在变量 `wv` 中,基因名称存储在变量 `vocabulary` 中。
3. `indexes` 是一个包含从0到 `wv` 的长度的列表,然后使用 `random.shuffle()` 方法对其进行随机打乱。
4. `topN` 是要保留的嵌入矩阵的前N个向量。
5. `rdWV` 是通过使用索引和切片操作从 `wv` 中选择前N个向量,并将其存储在新的变量中。
6. `rdVB` 是通过使用索引和切片操作从 `vocabulary` 中选择前N个基因名称,并将其存储在新的变量中。
7. 代码中的 `print("PCA!")` 和 `print("tsne!")` 是为了在控制台输出一些信息。
8. 使用 `PCA` 类对 `rdWV` 进行主成分分析降维,将维度减少为50,并将结果存储在 `pca_rdWV` 中。
9. 使用 `open()` 函数创建一个名为 "TSNE_label_gene2vec.txt" 的文件,并将 `rdVB` 中的基因名称逐行写入该文件。
10. `TSNEWoker` 函数用于执行TSNE操作。它接受一个参数 `iter`,并创建一个 `TSNE` 类的实例,指定了一些参数。然后使用 `fit_transform()` 方法对 `pca_rdWV` 进行TSNE降维,并将结果保存到文件 "TSNE_data_gene2vec.txt_"+iter+".txt" 中。
11. `mp_handler` 函数使用 `Pool` 类创建一个进程池,并调用 `p.map()` 方法将 `TSNEWoker` 函数应用于不同的迭代次数。
12. 最后,通过检查是否是主程序来执行代码。在这里,我们调用了 `mp_handler()` 函数,以并行方式生成不同迭代次数的TSNE可视化。
帮我用bert和pytorch等价实现nn.Embedding.from_pretrained()
当使用BERT和PyTorch时,可以使用以下代码实现nn.Embedding.from_pretrained()的等效功能:
```
import torch
from transformers import BertModel
# Load pre-trained BERT model
model = BertModel.from_pretrained('bert-base-uncased')
# Get the embedding weights from the BERT model
embedding_weights = model.embeddings.word_embeddings.weight
# Create an nn.Embedding layer with the pre-trained weights
embedding_layer = torch.nn.Embedding.from_pretrained(embedding_weights)
```
这将使用BERT模型中的预训练权重创建一个nn.Embedding层。
阅读全文
相关推荐
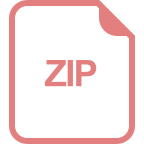
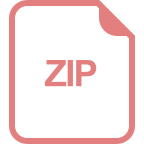
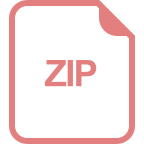









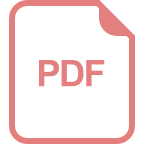
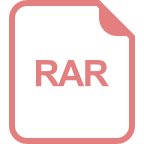
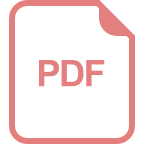
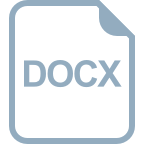
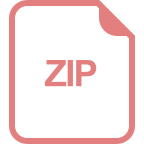