Java 分段配置文件
时间: 2023-06-10 20:06:56 浏览: 159
在 Java 中,可以使用 Properties 类来读取和写入配置文件。如果你需要将配置文件拆分成多个部分,可以考虑使用多个配置文件,或者使用一个主配置文件和多个附加配置文件。以下是一些示例代码,以便你更好地理解如何使用 Properties 类来实现分段配置文件。
1. 使用多个配置文件:
假设你有三个部分的配置文件:config1.properties,config2.properties和config3.properties。可以分别读取这些文件,然后将它们合并到一个 Properties 对象中,以便在代码中使用。
```java
Properties prop = new Properties();
try (InputStream input1 = new FileInputStream("config1.properties");
InputStream input2 = new FileInputStream("config2.properties");
InputStream input3 = new FileInputStream("config3.properties")) {
prop.load(input1);
prop.load(input2);
prop.load(input3);
} catch (IOException ex) {
ex.printStackTrace();
}
String value1 = prop.getProperty("key1");
String value2 = prop.getProperty("key2");
String value3 = prop.getProperty("key3");
```
2. 使用一个主配置文件和多个附加配置文件:
假设你有一个主配置文件 main.properties,其中包含了多个附加配置文件的名称,可以通过读取主配置文件,然后逐个读取附加配置文件,将它们合并到一个 Properties 对象中。
```java
Properties prop = new Properties();
try (InputStream input = new FileInputStream("main.properties")) {
prop.load(input);
String[] filenames = prop.getProperty("filenames").split(",");
for (String filename : filenames) {
try (InputStream input2 = new FileInputStream(filename)) {
prop.load(input2);
} catch (IOException ex) {
ex.printStackTrace();
}
}
} catch (IOException ex) {
ex.printStackTrace();
}
String value1 = prop.getProperty("key1");
String value2 = prop.getProperty("key2");
String value3 = prop.getProperty("key3");
```
在这种方法中,主配置文件 main.properties 应该包含类似于以下内容的行:
```
filenames=config1.properties,config2.properties,config3.properties
```
这些示例代码可以帮助你实现分段配置文件的功能。但是,在实际开发中,你可能需要更复杂的配置文件结构,并且需要编写更多的代码来处理它们。
阅读全文
相关推荐
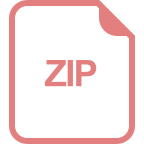


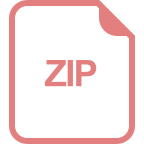
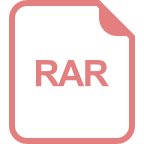
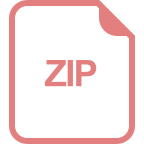
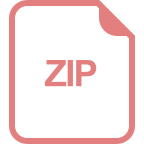
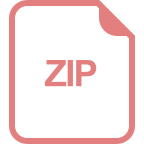
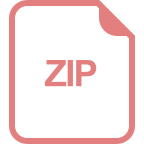
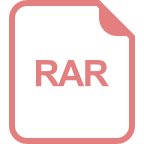
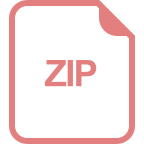
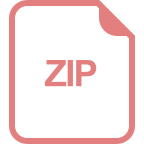
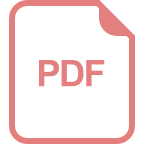
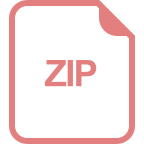
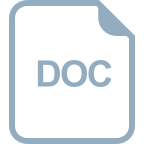
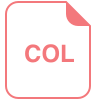