要求实现以下结构体或者函数(每个函数功能请严格按照要 求完成,不要修改,检查时会用统一main函数调用执行): struct Node; int list_tail_insert(Node *list_head, int var) // 单个数据插入,尾插法 Node *list_head_insert(Node *list_head, int var) //单个数据插入,头插法 Node *list_specific_insert(Node *list_head, int location, int var) //指定位置插入,可以插入头,尾, 或者头尾之间任意位置 void print_list(Node *list_head) //输出链表,循环 方式,空格隔开 void reverse_print_list(Node *list_head) //逆序输 出,递归方式,空格隔开 void change_specific_var(Node *list_head, int old_var, int new_var) //修改链表中的指定元素值 Node *del_specific_var(Node *list_head, int del_var) //删除链表中的指定元素值 Node *sort(Node *list_head) //从小到大排序 示例main函数中调用顺序 Node *list_head_insert Node *list_head_insert Node *list_head_insert int list_tail_insert int list_tail_insert Node *list_specific_insert void print_list Node *sort void print_listnvoid reverse_print_list void change_specific_var 2 3 void print_list Node *del_specific_var 3 void print_list
时间: 2023-05-19 18:01:42 浏览: 108
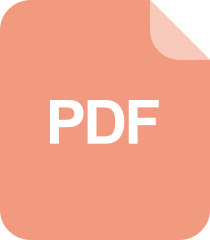
结构体类型数据作为函数参数(三种方法)
以下是实现要求的结构体和函数:
struct Node {
int val;
Node *next;
};
int list_tail_insert(Node *list_head, int var) {
Node *new_node = new Node;
new_node->val = var;
new_node->next = NULL;
if (list_head == NULL) {
list_head = new_node;
} else {
Node *cur = list_head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = new_node;
}
return 0;
}
Node *list_head_insert(Node *list_head, int var) {
Node *new_node = new Node;
new_node->val = var;
new_node->next = list_head;
return new_node;
}
Node *list_specific_insert(Node *list_head, int location, int var) {
Node *new_node = new Node;
new_node->val = var;
if (location == 0) {
new_node->next = list_head;
return new_node;
}
Node *cur = list_head;
for (int i = 0; i < location - 1; i++) {
cur = cur->next;
}
new_node->next = cur->next;
cur->next = new_node;
return list_head;
}
void print_list(Node *list_head) {
Node *cur = list_head;
while (cur != NULL) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
}
void reverse_print_list(Node *list_head) {
if (list_head == NULL) {
return;
}
reverse_print_list(list_head->next);
cout << list_head->val << " ";
}
void change_specific_var(Node *list_head, int old_var, int new_var) {
Node *cur = list_head;
while (cur != NULL) {
if (cur->val == old_var) {
cur->val = new_var;
}
cur = cur->next;
}
}
Node *del_specific_var(Node *list_head, int del_var) {
Node *dummy = new Node;
dummy->next = list_head;
Node *cur = dummy;
while (cur->next != NULL) {
if (cur->next->val == del_var) {
Node *temp = cur->next;
cur->next = cur->next->next;
delete temp;
} else {
cur = cur->next;
}
}
return dummy->next;
}
Node *sort(Node *list_head) {
if (list_head == NULL || list_head->next == NULL) {
return list_head;
}
Node *slow = list_head;
Node *fast = list_head->next;
while (fast != NULL && fast->next != NULL) {
slow = slow->next;
fast = fast->next->next;
}
Node *mid = slow->next;
slow->next = NULL;
Node *left = sort(list_head);
Node *right = sort(mid);
Node *dummy = new Node;
Node *cur = dummy;
while (left != NULL && right != NULL) {
if (left->val < right->val) {
cur->next = left;
left = left->next;
} else {
cur->next = right;
right = right->next;
}
cur = cur->next;
}
cur->next = (left == NULL) ? right : left;
return dummy->next;
}
示例main函数中调用顺序:
Node *list = NULL;
list = list_head_insert(list, 1);
list = list_head_insert(list, 2);
list = list_head_insert(list, 3);
list_tail_insert(list, 4);
list_tail_insert(list, 5);
list = list_specific_insert(list, 2, 6);
print_list(list);
list = sort(list);
print_list(list);
reverse_print_list(list);
change_specific_var(list, 2, 3);
print_list(list);
list = del_specific_var(list, 3);
print_list(list);
阅读全文
相关推荐
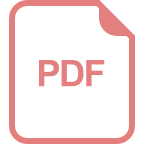
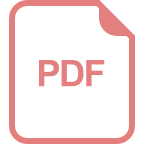















