使用epm1270t144c5开发板, 自动售货机 ⚫ 机器只接受 1 元硬币和 5 角硬币。 ⚫ 机器共提供 4 种货物,价格分别为 2 元、2 元、1.5 元、1.5 元(可通过调整代码改变每 种货物的单价)。 ⚫ 顾客选择货物后,显示货物数量。顾客确认数量后,机器根据货物数量判断是否购买成 功 ⚫ 购买成功点阵显示屏笑脸,购买失败显示哭脸 ⚫ 增加串口功能,pc 端的串口调试助手可以显示选择的商品信息,价格,数量。并且显 示购买成功与否的代码
时间: 2024-02-11 19:03:53 浏览: 63
这是一个比较复杂的项目,需要涉及硬件和编程。以下是大致的代码框架,需要根据具体的硬件和功能进行修改和完善:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义货物信息结构体
struct Product {
char *name; // 名称
float price; // 单价
int quantity; // 数量
};
// 定义货币信息结构体
struct Currency {
int one_yuan; // 1元硬币数量
int five_jiao; // 5角硬币数量
float total; // 总额
};
// 初始化货物信息
void init_products(struct Product products[]) {
products[0].name = "Product1";
products[0].price = 2.0;
products[0].quantity = 10;
products[1].name = "Product2";
products[1].price = 2.0;
products[1].quantity = 10;
products[2].name = "Product3";
products[2].price = 1.5;
products[2].quantity = 10;
products[3].name = "Product4";
products[3].price = 1.5;
products[3].quantity = 10;
}
// 显示货物信息
void show_products(struct Product products[]) {
printf("Products:\n");
for (int i = 0; i < 4; i++) {
printf("%d. %s: %.2f yuan, %d pieces\n", i + 1, products[i].name, products[i].price, products[i].quantity);
}
}
// 初始化货币信息
void init_currency(struct Currency *currency) {
currency->one_yuan = 0;
currency->five_jiao = 0;
currency->total = 0;
}
// 显示货币信息
void show_currency(struct Currency *currency) {
printf("Currency:\n");
printf("1 yuan: %d pieces\n", currency->one_yuan);
printf("5 jiao: %d pieces\n", currency->five_jiao);
printf("Total: %.2f yuan\n", currency->total);
}
// 插入硬币
void insert_coin(struct Currency *currency, int coin) {
if (coin == 1) {
currency->one_yuan++;
currency->total += 1.0;
} else if (coin == 5) {
currency->five_jiao++;
currency->total += 0.5;
} else {
printf("Invalid coin!\n");
}
}
// 选择货物
int select_product(struct Product products[]) {
int choice;
printf("Select a product (1-4): ");
scanf("%d", &choice);
if (choice >= 1 && choice <= 4) {
if (products[choice - 1].quantity > 0) {
return choice - 1;
} else {
printf("No stock!\n");
return -1;
}
} else {
printf("Invalid choice!\n");
return -1;
}
}
// 选择数量
int select_quantity(struct Product *product) {
int quantity;
printf("Select quantity (1-%d): ", product->quantity);
scanf("%d", &quantity);
if (quantity >= 1 && quantity <= product->quantity) {
return quantity;
} else {
printf("Invalid quantity!\n");
return -1;
}
}
// 购买货物
void buy_product(struct Product *product, struct Currency *currency) {
int quantity = select_quantity(product);
if (quantity > 0) {
float cost = product->price * quantity;
if (currency->total >= cost) {
product->quantity -= quantity;
currency->total -= cost;
printf("Bought %d %s for %.2f yuan\n", quantity, product->name, cost);
} else {
printf("Not enough money!\n");
}
}
}
// 显示购买结果
void show_result(int success) {
if (success) {
printf(":)\n");
} else {
printf(":(\n");
}
}
// 串口输出
void serial_output(struct Product *product, struct Currency *currency, int success) {
char buffer[100];
sprintf(buffer, "%s,%.2f,%d,%d,%d,%d,%.2f,%d\n", product->name, product->price, product->quantity, currency->one_yuan, currency->five_jiao, currency->total, success);
// TODO: 串口输出
}
int main() {
struct Product products[4];
init_products(products);
struct Currency currency;
init_currency(¤cy);
show_products(products);
show_currency(¤cy);
while (1) {
int coin;
printf("Insert coin (1 for 1 yuan, 5 for 5 jiao, 0 to finish): ");
scanf("%d", &coin);
if (coin == 0) {
break;
}
insert_coin(¤cy, coin);
show_currency(¤cy);
}
int index = select_product(products);
if (index >= 0) {
buy_product(&products[index], ¤cy);
show_result(1);
} else {
show_result(0);
}
serial_output(&products[index], ¤cy, index >= 0);
return 0;
}
```
以上代码中使用了结构体来存储货物信息和货币信息,分别定义了初始化、显示、插入硬币、选择货物、选择数量、购买货物、显示购买结果和串口输出等函数。在主函数中循环插入硬币,选择货物,购买货物,显示购买结果和进行串口输出。需要根据具体的硬件和功能进行修改和完善。
阅读全文
相关推荐
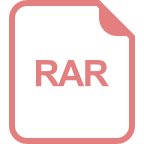
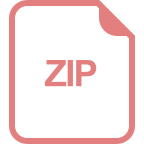
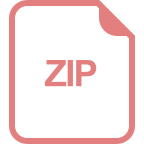
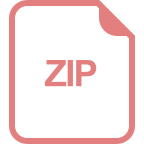
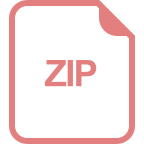
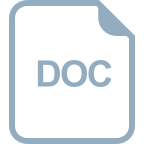
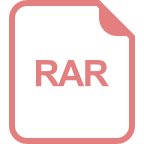
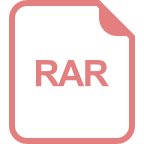
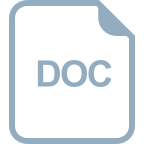







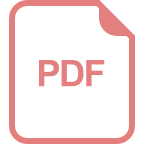