在实验板8*8点阵稳定显示红色心形图案,实验板输入时钟选50MHz,编写quartus的vhdl代码,给出电路逻辑结构图的设计思路(实验板用EPM1270T144C5芯片)
时间: 2023-06-24 19:05:48 浏览: 129
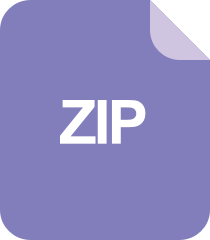
(练习)使用vhdl编写的组合逻辑 时钟.zip

首先,我们需要将心形图案转换为8x8的点阵图案。然后,我们需要将点阵图案存储在芯片内部的存储器中。接着,我们需要编写 VHDL 代码,通过时钟信号来控制点阵图案的显示。最后,我们需要将输出信号连接到实验板上的 LED 灯上,以便观察。
下面是一个简单的设计思路:
1. 使用 MATLAB 或其他图像处理软件,将心形图案转换为一个8x8的点阵图案。将每个像素点对应到一个二进制位上,0表示灭,1表示亮。
2. 将点阵图案存储在芯片内部的存储器中。可以使用 ROM 存储器或 LUT 存储器来实现。存储器的地址由一个计数器控制,计数器的值逐个递增,直到达到63为止。
3. 编写 VHDL 代码来控制点阵图案的显示。代码应该包括一个计数器和一个选择器。计数器用于逐个递增地访问存储器的地址,选择器用于选择存储器中的数据,并将数据输出到 LED 灯上。
4. 将 VHDL 代码合成为电路逻辑结构图,并将输出信号连接到实验板上的 LED 灯上。可以使用 Quartus 软件来完成这个过程。
需要注意的是,由于实验板的时钟频率为50MHz,因此需要在 VHDL 代码中使用分频器来将时钟频率降低到合适的水平,以便正确控制 LED 灯的闪烁频率。
阅读全文
相关推荐
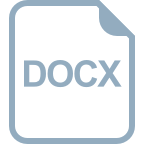

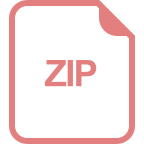
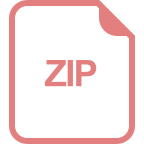
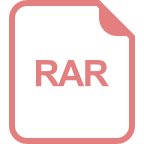
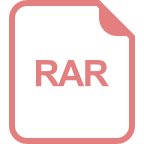
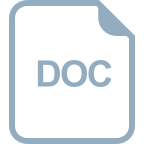
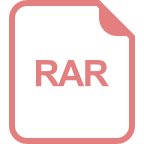
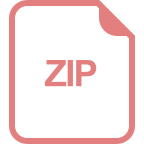
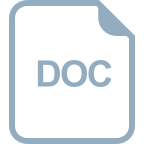
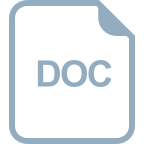
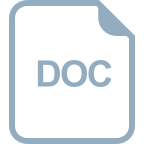
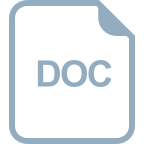
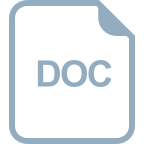
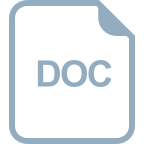
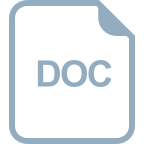