使用Java编写一个对线程的调用
时间: 2024-01-21 19:19:10 浏览: 25
下面是一个简单的Java程序,用于演示如何调用线程:
```java
public class ThreadExample implements Runnable {
private Thread thread;
private String threadName;
ThreadExample(String name) {
threadName = name;
System.out.println("Creating " + threadName);
}
public void run() {
System.out.println("Running " + threadName);
try {
for (int i = 4; i > 0; i--) {
System.out.println("Thread: " + threadName + ", " + i);
Thread.sleep(50);
}
} catch (InterruptedException e) {
System.out.println("Thread " + threadName + " interrupted.");
}
System.out.println("Thread " + threadName + " exiting.");
}
public void start() {
System.out.println("Starting " + threadName);
if (thread == null) {
thread = new Thread(this, threadName);
thread.start();
}
}
public static void main(String[] args) {
ThreadExample example1 = new ThreadExample("Thread 1");
example1.start();
ThreadExample example2 = new ThreadExample("Thread 2");
example2.start();
}
}
```
这个程序创建了一个名为 `ThreadExample` 的类,它实现了 `Runnable` 接口。在这个类中,我们定义了一个 `Thread` 对象和一个线程名字。我们还实现了 `run()` 方法,在其中编写了线程要执行的代码。在 `start()` 方法中,我们创建了一个新的线程并启动它。
在 `main()` 方法中,我们创建了两个 `ThreadExample` 对象,并调用它们的 `start()` 方法。这将启动两个线程并开始执行它们的 `run()` 方法。
输出结果如下:
```
Creating Thread 1
Starting Thread 1
Creating Thread 2
Starting Thread 2
Running Thread 1
Thread: Thread 1, 4
Running Thread 2
Thread: Thread 2, 4
Thread: Thread 1, 3
Thread: Thread 2, 3
Thread: Thread 1, 2
Thread: Thread 2, 2
Thread: Thread 1, 1
Thread: Thread 2, 1
Thread Thread 1 exiting.
Thread Thread 2 exiting.
```
可以看到,两个线程交替执行,每个线程都打印了一些消息,并在最后退出。
相关推荐
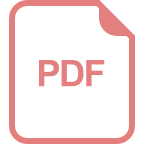
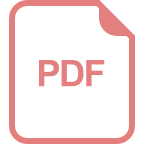
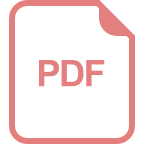














