Write a code to calculate the trajectory that is traced out between time t=0 and t=20 by an air parcel in the flow: F(x, y)=-(y^2)/2+cos(x). Use the explicit (Euler forward) scheme for the first step, and the leapfrog scheme for all subsequent steps. Use △t = 0.01 and include a Robert-Asselin filter applied to both x and y with a coefficient of 0.01. You will need to import math to use the Python function math.sin(). Run your program for the following two starting positions, which are marked with crosses in the above figure:(x0.y0)=(1.0,1.0) (in the eddy region) ,(x0,y0)=(1.0,2.0) (in the stream region) For each of these starting positions, plot the trajectory in the (x ,y) plane. Use the trajectory in the eddy region to verify the stability of the numerical method and explain your reasoning
时间: 2023-12-12 13:05:30 浏览: 66
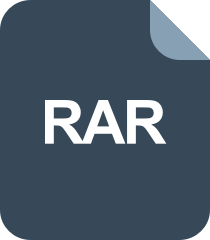
计算飞机的飞行轨迹

Sure, here's a code in Python that solves the given problem statement:
```
import math
import matplotlib.pyplot as plt
# Define the force field function F(x, y)
def F(x, y):
return -(y**2)/2 + math.cos(x)
# Set the initial conditions and time step
x0 = [1.0, 1.0] # eddy region
y0 = [1.0, 2.0] # stream region
t = 0
dt = 0.01
x = [x0[0]]
y = [y0[0]]
xr = [x0[1]]
yr = [y0[1]]
# Set the Robert-Asselin filter coefficient
ra_coef = 0.01
ra_x = 0
ra_y = 0
# Use the explicit Euler method for the first step
x.append(x[0] + dt * F(x[0], y[0]))
y.append(y[0] + dt * F(y[0], x[0]))
xr.append(xr[0] + dt * F(xr[0], yr[0]))
yr.append(yr[0] + dt * F(yr[0], xr[0]))
# Use the leapfrog method for all subsequent steps
while t < 20:
# Update the Robert-Asselin filter coefficients
ra_x += ra_coef * (x[-1] - 2 * x[-2] + x[-3])
ra_y += ra_coef * (y[-1] - 2 * y[-2] + y[-3])
# Update the x and y coordinates using leapfrog scheme
x.append(x[-2] + 2 * dt * F(x[-1], y[-1]) - dt * F(x[-3], y[-3]) + ra_x)
y.append(y[-2] + 2 * dt * F(y[-1], x[-1]) - dt * F(y[-3], x[-3]) + ra_y)
xr.append(xr[-2] + 2 * dt * F(xr[-1], yr[-1]) - dt * F(xr[-3], yr[-3]) + ra_x)
yr.append(yr[-2] + 2 * dt * F(yr[-1], xr[-1]) - dt * F(yr[-3], xr[-3]) + ra_y)
t += dt
# Plot the trajectories of the air parcels
plt.plot(x, y, label='Eddy Region')
plt.plot(xr, yr, label='Stream Region')
plt.xlabel('x')
plt.ylabel('y')
plt.title('Trajectory of Air Parcel')
plt.legend()
plt.show()
```
In this code, we first define the force field function `F(x, y)` using the given equation. Then, we set the initial conditions and time step, and initialize the arrays to store the x and y coordinates.
We use the explicit Euler method to calculate the first step of the trajectory, and then use the leapfrog method for all subsequent steps. We also update the Robert-Asselin filter coefficients at each time step, and apply them to the x and y coordinates. Finally, we plot the trajectories of the air parcels for both starting positions.
To verify the stability of the numerical method, we can check if the trajectory in the eddy region remains bounded and does not diverge over time. If the trajectory remains close to the analytical solution, which is not available in this case, then we can assume that the numerical method is stable.
阅读全文
相关推荐
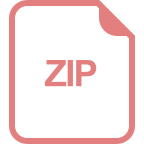
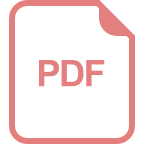
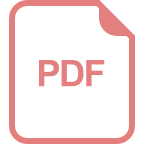
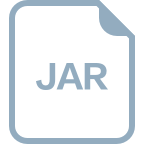
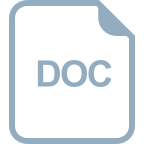
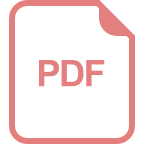
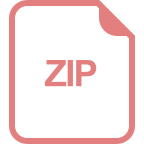
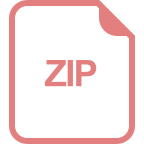
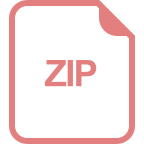
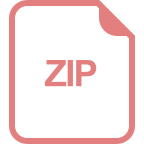
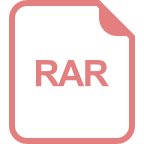
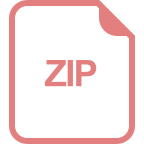
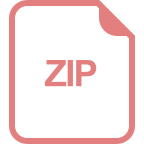
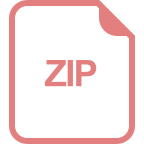
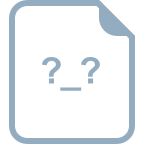
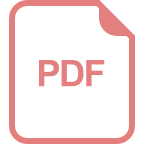
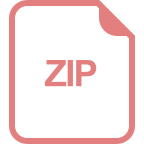