db.execSQL("select * from user where name ="+name);
时间: 2024-02-21 18:57:49 浏览: 30
在执行SQL语句时,应该使用`query()`或`rawQuery()`方法,而不是`execSQL()`方法。`execSQL()`方法用于执行不返回结果集的SQL语句,例如CREATE、UPDATE、DELETE等语句,而`query()`或`rawQuery()`方法用于执行SELECT查询语句并返回结果集。另外,你应该使用参数化查询,避免SQL注入攻击。正确的写法如下:
```
Cursor cursor = db.rawQuery("SELECT * FROM user WHERE name = ?", new String[]{name});
```
这个语句使用了`rawQuery()`方法,并采用了参数化查询的方式,其中`?`表示占位符,`new String[]{name}`表示将`name`作为参数传入SQL语句中。这样可以确保查询语句的正确性,并避免SQL注入攻击。
相关问题
db.execSQL("update user set Name=? ,Nickname=?,Age=?",new Object[]{name,nickname,age}); Cursor cursor= db.rawQuery("select*from user where Account=?",new String[]{account}); editTextname.setText(cursor.getString(2)); editTextnickname.setText(cursor.getString(3)); editTextage.setText(cursor.getString(4)); cursor.close();显示更新的数据哪错了
这段代码有几个问题:
1. `update` 语句中的参数应该是按照字段的顺序传递的,而不是按照字段名称。因此,应该将 `Name`、`Nickname`、`Age` 改为对应的字段顺序(假设是第 2、3、4 个字段)。
2. 在执行 `rawQuery` 方法时,需要先调用 `moveToFirst` 方法将结果集指针移动到第一条记录上,然后才能获取数据。因此,在调用 `cursor.getString` 方法之前,应该先调用 `cursor.moveToFirst` 方法。
修改后的代码应该是这样的:
```
db.execSQL("update user set ?, ?, ? where Account=?", new Object[]{name, nickname, age, account});
Cursor cursor = db.rawQuery("select * from user where Account=?", new String[]{account});
if (cursor.moveToFirst()) {
editTextname.setText(cursor.getString(2));
editTextnickname.setText(cursor.getString(3));
editTextage.setText(cursor.getString(4));
}
cursor.close();
```
from PyQt5 import QtWidgets, QtSql import sys class Login(QtWidgets.QWidget): def __init__(self): super().__init__() self.init_ui() def init_ui(self): # 创建控件 self.username = QtWidgets.QLineEdit() self.password = QtWidgets.QLineEdit() self.password.setEchoMode(QtWidgets.QLineEdit.Password) self.login_btn = QtWidgets.QPushButton('登录') # 创建布局 layout = QtWidgets.QVBoxLayout() layout.addWidget(QtWidgets.QLabel('用户名')) layout.addWidget(self.username) layout.addWidget(QtWidgets.QLabel('密码')) layout.addWidget(self.password) layout.addWidget(self.login_btn) # 设 置布局 self.setLayout(layout) # 链接数据库 db = QtSql.QSqlDatabase.addDatabase('QSQLITE') db.setDatabaseName('test.db') if not db.open(): QtWidgets.QMessageBox.critical(None, "无法打开数据库", "无法建立到数据库的连接,这个例子需要SQLite支 持,请检查数据库配置\n\n" "点击取消退出应用程序", QtWidgets. QMessageBox.Cancel) return # 创建表格 query = QtSql.QSqlQuery() query.exec_("create table user (id int primary key, username varchar(20), password varchar(20))") query.exec_("insert into user values(1, 'admin', 'admin')") # 链接信号和槽 self.login_btn.clicked.connect(self.login) def login(self): username = self.username.text().strip() password = self.password.text().strip() # 验证用户名和密码 query = QtSql.QSqlQuery() sql = "select * from user where username='{0}' and password='{1}'".format(username, password) query.exec_(sql) if query.next(): QtWidgets.QMessageBox.information(None, '登录成功 ', '登录成功') else: QtWidgets.QMessageBox.warning(None, '登录失败', '用户名或密码错误') if __name__ == '__main__': app = QtWidgets.QApplication(sys.argv) window = Login() window.show() sys.exit(app.exec_())
这是一个使用PyQt5连接SQLite数据库实现登录功能的示例代码。代码中通过创建控件、布局以及链接信号和槽等方式实现了一个简单的登录界面。其中,通过QtSql模块连接SQLite数据库,并创建了一个名为"user"的表格,用于存储用户信息。在登录时,通过查询用户输入的用户名和密码是否在数据库中存在来验证用户身份,并弹出相应的提示框。
相关推荐
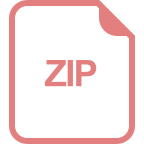
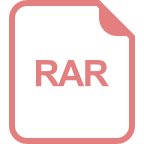
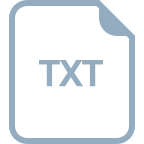












