java中如何用sm4加密一个文件
时间: 2024-05-17 22:17:11 浏览: 163
在Java中使用SM4加密文件的过程包括以下几个步骤:
1. 读取原始文件内容,将其存储到一个字节数组中。
2. 生成SM4加密密钥,使用SM4算法对文件内容进行加密。
3. 将加密后的内容存储到一个新的文件中。
下面是一个使用Bouncy Castle库实现的SM4加密文件的示例代码:
```java
import org.bouncycastle.crypto.engines.SM4Engine;
import org.bouncycastle.crypto.params.KeyParameter;
import org.bouncycastle.crypto.params.ParametersWithIV;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.nio.charset.StandardCharsets;
import java.security.Security;
public class SM4FileEncryption {
private static final int BLOCK_SIZE = 16;
private static final String ALGORITHM = "SM4/ECB/PKCS7Padding";
private static final byte[] IV = new byte[BLOCK_SIZE];
public static void main(String[] args) throws Exception {
Security.addProvider(new BouncyCastleProvider());
File inputFile = new File("plain.txt");
File outputFile = new File("cipher.txt");
byte[] key = "0123456789abcdef".getBytes(StandardCharsets.UTF_8);
encryptFile(inputFile, outputFile, key);
}
private static void encryptFile(File inputFile, File outputFile, byte[] key) throws Exception {
byte[] input = new byte[(int) inputFile.length()];
try (FileInputStream fis = new FileInputStream(inputFile)) {
fis.read(input);
}
Cipher cipher = Cipher.getInstance(ALGORITHM, "BC");
SecretKeySpec keySpec = new SecretKeySpec(key, "SM4");
cipher.init(Cipher.ENCRYPT_MODE, keySpec);
byte[] output = new byte[cipher.getOutputSize(input.length)];
int outputLength = cipher.update(input, 0, input.length, output, 0);
outputLength += cipher.doFinal(output, outputLength);
try (FileOutputStream fos = new FileOutputStream(outputFile)) {
fos.write(output, 0, outputLength);
}
}
}
```
在上述代码中,我们使用Bouncy Castle库提供的Cipher类进行SM4加密,其中`SM4/ECB/PKCS7Padding`表示采用ECB模式和PKCS7填充方式。密钥和输入文件从文件中读取,加密后的结果存储到输出文件中。需要注意的是,SM4算法是分块加密算法,因此要对加密内容进行分块处理,本示例采用的是`Cipher.getOutputSize()`方法进行分块处理。
另外,你也可以使用Bouncy Castle库提供的SM4Engine类进行加密。具体示例代码可参考我在前面的回答中提供的示例。
阅读全文
相关推荐
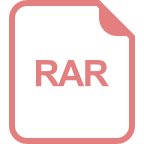
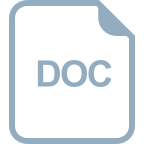










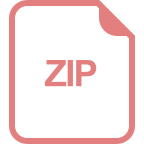