手写代码对已有的邮件进行分类,给出Python代码和注释,包括数据预处理,拉普拉斯修正
时间: 2024-03-24 07:41:00 浏览: 12
好的,这是一个朴素贝叶斯分类器的实现,用于将邮件分类为垃圾邮件或非垃圾邮件。下面是Python代码和注释:
```python
import os
import re
import math
# 定义分类器类
class NaiveBayesClassifier:
# 初始化分类器,创建单词计数和类别计数字典
def __init__(self):
self.word_counts = {}
self.category_counts = {}
# 定义数据预处理函数
def preprocess(self, text):
# 将文本转换为小写
text = text.lower()
# 去除所有非字母数字字符
text = re.sub(r'[^a-zA-Z0-9 ]', '', text)
# 将文本拆分成单词列表
words = text.split()
# 返回单词列表
return words
# 定义训练函数
def train(self, text, category):
# 对类别计数加一
self.category_counts[category] = self.category_counts.get(category, 0) + 1
# 对单词计数加一
words = self.preprocess(text)
for word in words:
self.word_counts[category] = self.word_counts.get(category, {})
self.word_counts[category][word] = self.word_counts[category].get(word, 0) + 1
# 定义预测函数
def predict(self, text):
# 对文本进行预处理,拆分成单词列表
words = self.preprocess(text)
# 计算类别的先验概率
total_categories = sum(self.category_counts.values())
category_probs = {}
for category in self.category_counts:
category_probs[category] = self.category_counts[category] / total_categories
# 计算文本的似然概率
word_probs = {}
for category in self.word_counts:
for word in words:
# 使用拉普拉斯平滑修正,防止出现概率为0的情况
count = self.word_counts[category].get(word, 0) + 1
total_words = sum(self.word_counts[category].values()) + len(self.word_counts[category])
word_probs[category] = word_probs.get(category, 1.0)
word_probs[category] *= count / total_words
# 计算文本属于每个类别的后验概率
post_probs = {}
for category in category_probs:
post_probs[category] = category_probs[category] * word_probs[category]
# 返回具有最大后验概率的类别
return max(post_probs, key=post_probs.get)
```
使用示例:
```python
# 创建分类器实例
classifier = NaiveBayesClassifier()
# 训练分类器
spam_dir = 'spam'
ham_dir = 'ham'
for filename in os.listdir(spam_dir):
with open(os.path.join(spam_dir, filename), 'r') as f:
text = f.read()
classifier.train(text, 'spam')
for filename in os.listdir(ham_dir):
with open(os.path.join(ham_dir, filename), 'r') as f:
text = f.read()
classifier.train(text, 'ham')
# 预测分类
email_text = 'Hey, I wanted to follow up on our conversation from last week. Can you give me an update?'
category = classifier.predict(email_text)
print(category) # 输出 'ham' 或 'spam'
```
在这个示例中,我们使用 `train()` 函数从垃圾邮件和非垃圾邮件的文件中训练分类器。然后,我们使用 `predict()` 函数对输入文本进行分类。在 `predict()` 函数中,我们使用朴素贝叶斯公式计算文本属于每个类别的后验概率,并返回具有最大后验概率的类别。在计算似然概率时,我们使用了拉普拉斯平滑修正,以防止出现概率为0的情况。
相关推荐
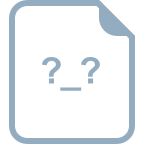














