vue3+springboot2实现登录注册代码
时间: 2024-01-31 22:04:01 浏览: 23
以下是一个基于Vue3和Spring Boot2的简单登录/注册示例代码:
前端代码(Vue3):
```html
<template>
<div>
<h1>登录</h1>
<form>
<label for="username">用户名:</label>
<input type="text" v-model="username" name="username" required>
<label for="password">密码:</label>
<input type="password" v-model="password" name="password" required>
<button @click.prevent="login">登录</button>
</form>
<h1>注册</h1>
<form>
<label for="username">用户名:</label>
<input type="text" v-model="newUser.username" name="username" required>
<label for="email">邮箱:</label>
<input type="email" v-model="newUser.email" name="email" required>
<label for="password">密码:</label>
<input type="password" v-model="newUser.password" name="password" required>
<button @click.prevent="register">注册</button>
</form>
</div>
</template>
<script>
import { ref } from 'vue';
export default {
name: 'LoginRegister',
setup() {
const username = ref('');
const password = ref('');
const newUser = ref({
username: '',
password: '',
email: ''
});
const login = () => {
// 发送登录请求
const data = { username: username.value, password: password.value };
// 使用axios或其他HTTP库发送POST请求到后端API
// ...
};
const register = () => {
// 发送注册请求
// 使用axios或其他HTTP库发送POST请求到后端API
// ...
};
return {
username,
password,
newUser,
login,
register
};
},
};
</script>
```
后端代码(Spring Boot2):
```java
@RestController
@RequestMapping("/api/auth")
public class AuthController {
@Autowired
private AuthenticationManager authenticationManager;
@Autowired
private JwtTokenUtil jwtTokenUtil;
@Autowired
private UserDetailsService userDetailsService;
@PostMapping("/login")
public ResponseEntity<?> login(@RequestBody LoginRequest request) {
final Authentication authentication = authenticationManager.authenticate(
new UsernamePasswordAuthenticationToken(request.getUsername(), request.getPassword()));
SecurityContextHolder.getContext().setAuthentication(authentication);
final UserDetails userDetails = userDetailsService.loadUserByUsername(request.getUsername());
final String token = jwtTokenUtil.generateToken(userDetails);
return ResponseEntity.ok(new JwtResponse(token));
}
@PostMapping("/register")
public ResponseEntity<?> register(@RequestBody SignupRequest request) {
User user = new User(request.getUsername(), request.getEmail(), request.getPassword());
return ResponseEntity.ok(userDetailsService.save(user));
}
}
```
其中,`LoginRequest`和`SignupRequest`分别是登录和注册请求的数据模型,`JwtTokenUtil`用于生成和验证JWT Token,`UserDetailsService`用于从数据库中加载用户信息。在这个示例中,我们使用了Spring Security进行身份验证,使用JWT Token进行API安全性保护。当然,这仅仅是一个简单示例,实际应用中还需要考虑很多其他的安全问题。
相关推荐
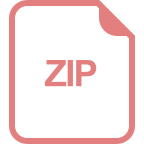
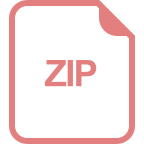
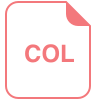













