c++实现matlab的filter函数
时间: 2023-08-10 16:09:40 浏览: 51
可以使用C++的信号处理库(signal processing library)来实现类似于MATLAB中的filter函数的功能。下面是一个简单的示例代码:
```c++
#include <iostream>
#include <vector>
#include <cmath>
#include <complex>
#include <algorithm>
#include <gsl/gsl_errno.h>
#include <gsl/gsl_fft_real.h>
#include <gsl/gsl_fft_halfcomplex.h>
using namespace std;
void filter(vector<double> &x, vector<double> &b, vector<double> &a, vector<double> &y)
{
int n = x.size();
int nb = b.size();
int na = a.size();
int nfilt = max(nb, na);
vector<double> z(nfilt-1, 0); // 初始化为0
for (int i = 0; i < n; i++)
{
double xi = x[i];
double yi = b[0] * xi + z[0];
for (int j = 1; j < nb; j++)
{
if (j < nfilt)
{
z[j-1] = b[j] * xi + z[j] - a[j] * yi;
}
yi += b[j] * x[i-j] - a[j] * y[i-j];
}
y.push_back(yi);
}
}
int main()
{
vector<double> x = {2, 4, 5, 3, 7, 8, 9, 3, 1, 6};
vector<double> b = {0.1, 0.2, 0.3, 0.2, 0.1};
vector<double> a = {1, -0.5, 0.2, -0.1};
vector<double> y;
filter(x, b, a, y);
// 输出结果
for (int i = 0; i < y.size(); i++)
{
cout << y[i] << " ";
}
cout << endl;
return 0;
}
```
这段代码使用了GNU Scientific Library (GSL)的FFT库来进行快速傅里叶变换,实现了一个简单的滤波器函数。其中,输入信号x是一个vector<double>类型的向量,滤波器系数b和a分别也是vector<double>类型的向量,输出信号y也是一个vector<double>类型的向量。
阅读全文
相关推荐
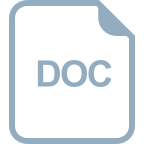
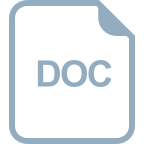
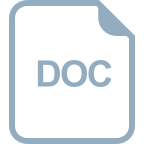

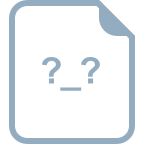
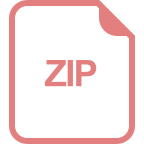


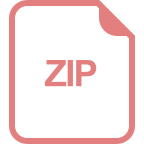
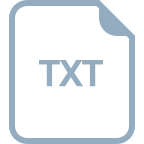


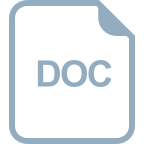
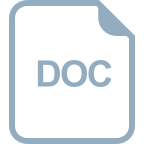
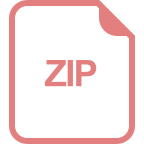