C语言输入年月,输出该月天数
时间: 2023-09-26 07:11:41 浏览: 446
可以以下的代码实现:
```c
#include <stdio.h>
int main() {
int year, month;
printf("请输入年份和月份(用空格分隔):\n");
scanf("%d %d", &year, &month);
int days;
switch (month) {
case 2:
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
days = 29;
} else {
days = 28;
}
break;
case 4:
case 6:
case 9:
case 11:
days = 30;
break;
default:
days = 31;
break;
}
printf("%d 年 %d 月共有 %d 天\n", year, month, days);
return 0;
}
```
这里使用了一个 `switch` 语句,根据输入的月份计算出该月有多少天。需要注意的是,闰年的二月份有29天,平年的二月份有28天。因此需要先判断是否为闰年。
相关问题
C语言用switch语句输入年月,输出该月天数
在C语言中,你可以使用`switch`语句配合数组或者一系列的`if...else if...else`结构来根据用户输入的月份判断并输出对应的天数。这里是一个简单的例子,假设我们只处理一年中的常规月份(非闰年):
```c
#include <stdio.h>
int main() {
int month, days_in_month;
printf("请输入月份(1-12): ");
scanf("%d", &month);
// 定义每个月份的天数
const int month_days[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
days_in_month = month_days[month - 1]; // 从一月开始计数
break; // 结束case,跳出switch
case 4:
case 6:
case 9:
case 11:
days_in_month = month_days[month - 2]; // 四月至十一月也是30天
break;
default:
printf("输入错误,月份无效!\n");
return 1; // 如果输入不是1到12,返回错误代码
}
printf("输入的月份 %d 有 %d 天。\n", month, days_in_month);
return 0;
}
```
c语言输入年月判断天数
根据提供的引用内容,没有直接回答C语言输入年月判断天数的代码。但是我们可以根据C语言的基本语法和逻辑来编写这样的代码。以下是一个简单的例子:
```c
#include <stdio.h>
int main() {
int year, month, days;
printf("请输入年份和月份:");
scanf("%d%d", &year, &month);
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
days = 31;
break;
case 4:
case 6:
case 9:
case 11:
days = 30;
break;
case 2:
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
days = 29;
} else {
days = 28;
}
break;
default:
printf("输入的月份有误!\n");
return 0;
}
printf("%d年%d月有%d天。\n", year, month, days);
return 0;
}
```
该程序首先要求用户输入年份和月份,然后根据月份的不同计算出该月的天数。其中,对于2月份需要特殊处理,判断是否为闰年。最后输出结果。
阅读全文
相关推荐
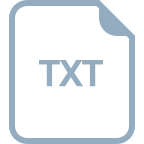
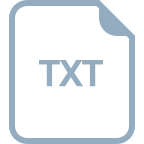











