非线性回归预测模型python
时间: 2024-01-26 14:14:39 浏览: 106
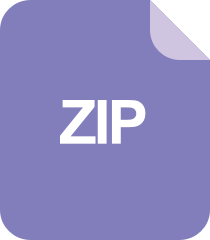
基于python实现的机器学习预测系统汇总+GUI界面(贝叶斯网络、马尔科夫模型、线性回归、岭回归多项式回归、决策树等).zip

非线性回归预测模型在Python中可以使用多种方法实现,其中包括BP神经网络和逻辑回归模型。下面我将分别介绍这两种方法的实现。
1. BP神经网络回归预测模型:
BP神经网络是一种常用的非线性回归预测模型,它可以通过训练来学习输入和输出之间的映射关系。在Python中,可以使用第三方库如TensorFlow、Keras或PyTorch来实现BP神经网络。
以下是一个使用Keras库实现BP神经网络回归预测模型的示例代码:
```python
import numpy as np
from keras.models import Sequential
from keras.layers import Dense
# 创建模型
model = Sequential()
model.add(Dense(10, input_dim=1, activation='relu'))
model.add(Dense(1, activation='linear'))
# 编译模型
model.compile(loss='mean_squared_error', optimizer='adam')
# 准备数据
X = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
y = np.array([2, 4, 6, 8, 10, 12, 14, 16, 18, 20])
# 训练模型
model.fit(X, y, epochs=1000, verbose=0)
# 预测
x_test = np.array([11, 12, 13, 14, 15])
y_pred = model.predict(x_test)
print("预测结果:", y_pred.flatten())
```
2. 基于Python的非线性回归(逻辑回归)模型:
逻辑回归是一种常用的非线性回归模型,它可以用于二分类或多分类问题。在Python中,可以使用第三方库如scikit-learn来实现逻辑回归模型。
以下是一个使用scikit-learn库实现逻辑回归模型的示例代码:
```python
from sklearn.linear_model import LogisticRegression
from sklearn.preprocessing import PolynomialFeatures
# 准备数据
X = [[1], [2], [3], [4], [5], [6], [7], [8], [9], [10]]
y = [0, 0, 0, 0, 1, 1, 1, 1, 1, 1]
# 特征转换
poly = PolynomialFeatures(degree=2)
X_poly = poly.fit_transform(X)
# 创建模型
model = LogisticRegression()
# 训练模型
model.fit(X_poly, y)
# 预测
x_test = [[11], [12], [13], [14], [15]]
x_test_poly = poly.transform(x_test)
y_pred = model.predict(x_test_poly)
print("预测结果:", y_pred)
```
阅读全文
相关推荐
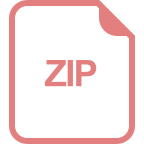
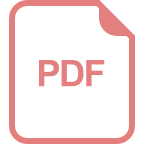


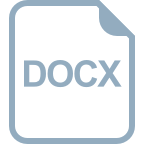
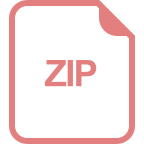
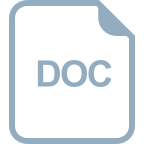
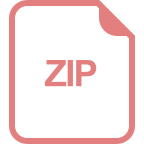
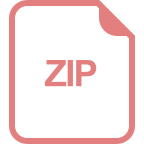
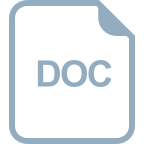
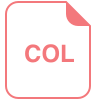




