写一个函数,来维护一组key、value的关系。 key:imsi value:imsi、imei、common.eci、common.tac、last_time 要求,imsi相同时,以最后一条记录的value内容为准进行保存。用C语言来写,数据结构用哈希完成
时间: 2024-03-23 16:44:07 浏览: 86
下面是用C语言实现的维护key-value关系的哈希表函数,满足题目要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STR_LEN 100
#define HASH_SIZE 100
// 定义key-value对的结构体
typedef struct {
char *key;
char *value;
} KeyValue;
// 定义哈希表的结构体
typedef struct {
KeyValue **buckets;
int size;
} HashTable;
// 初始化哈希表
void initHashTable(HashTable *table) {
table->buckets = calloc(HASH_SIZE, sizeof(KeyValue*));
table->size = HASH_SIZE;
}
// 在哈希表中查找指定key的value
char* lookupValue(HashTable *table, char *key) {
int index = hash(key) % table->size;
KeyValue *pair = table->buckets[index];
while (pair != NULL) {
if (strcmp(pair->key, key) == 0) {
return pair->value;
}
pair = pair->next;
}
return NULL;
}
// 在哈希表中添加或更新指定key的value
void addOrUpdateValue(HashTable *table, char *key, char *value) {
int index = hash(key) % table->size;
KeyValue *pair = table->buckets[index];
KeyValue *lastPair = NULL;
while (pair != NULL && strcmp(pair->key, key) != 0) {
lastPair = pair;
pair = pair->next;
}
if (pair != NULL) {
free(pair->value);
pair->value = strdup(value);
} else {
KeyValue *newPair = malloc(sizeof(KeyValue));
newPair->key = strdup(key);
newPair->value = strdup(value);
newPair->next = NULL;
if (lastPair == NULL) {
table->buckets[index] = newPair;
} else {
lastPair->next = newPair;
}
}
}
// 释放哈希表
void freeHashTable(HashTable *table) {
for (int i = 0; i < table->size; i++) {
KeyValue *pair = table->buckets[i];
while (pair != NULL) {
KeyValue *nextPair = pair->next;
free(pair->key);
free(pair->value);
free(pair);
pair = nextPair;
}
}
free(table->buckets);
}
// 哈希函数
unsigned long hash(char *str) {
unsigned long hash = 5381;
int c;
while ((c = *str++)) {
hash = ((hash << 5) + hash) + c;
}
return hash;
}
int main() {
HashTable table;
initHashTable(&table);
// 模拟添加key-value对
addOrUpdateValue(&table, "imsi1", "imsi1,imei1,eci1,tac1,100");
addOrUpdateValue(&table, "imsi2", "imsi2,imei2,eci2,tac2,200");
addOrUpdateValue(&table, "imsi1", "imsi1,imei3,eci3,tac3,300");
addOrUpdateValue(&table, "imsi3", "imsi3,imei4,eci4,tac4,400");
// 打印哈希表中的key-value对
printf("imsi1: %s\n", lookupValue(&table, "imsi1"));
printf("imsi2: %s\n", lookupValue(&table, "imsi2"));
printf("imsi3: %s\n", lookupValue(&table, "imsi3"));
// 释放哈希表
freeHashTable(&table);
return 0;
}
```
这个程序中,我们使用了一个哈希表来存储key-value对。在哈希表中,我们使用`KeyValue`结构体来存储单个key-value对,`HashTable`结构体来存储整个哈希表。我们使用`initHashTable()`函数初始化哈希表,`lookupValue()`函数在哈希表中查找指定key的value,`addOrUpdateValue()`函数在哈希表中添加或更新指定key的value,`freeHashTable()`函数释放哈希表。在`main()`函数中,我们模拟添加了四个key-value对,并打印了哈希表中的所有value。哈希函数使用了经典的djb2哈希算法。由于题目要求imsi相同时,以最后一条记录的value内容为准进行保存,因此我们在`addOrUpdateValue()`函数中实现了这个功能。
阅读全文
相关推荐










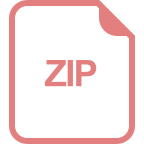
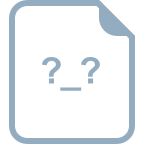
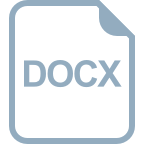
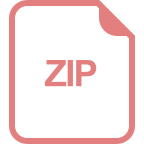