用结构体数组写个通讯录系统的c语言代码,包括增加、删除、修改、查找、保存的功能,里面储存联系人的姓名、性别、年龄、家庭电话、移动电话、办公电话
时间: 2023-09-19 15:12:14 浏览: 46
以下是一个简单的通讯录系统的 C 语言代码,使用结构体数组实现:
```c
#include <stdio.h>
#include <string.h>
#define MAX_CONTACTS 100
struct Contact {
char name[50];
char gender[10];
int age;
char home_phone[20];
char mobile_phone[20];
char office_phone[20];
};
struct Contact contacts[MAX_CONTACTS];
int num_contacts = 0;
void add_contact() {
struct Contact new_contact;
printf("Enter name: ");
scanf("%s", new_contact.name);
printf("Enter gender: ");
scanf("%s", new_contact.gender);
printf("Enter age: ");
scanf("%d", &new_contact.age);
printf("Enter home phone: ");
scanf("%s", new_contact.home_phone);
printf("Enter mobile phone: ");
scanf("%s", new_contact.mobile_phone);
printf("Enter office phone: ");
scanf("%s", new_contact.office_phone);
contacts[num_contacts++] = new_contact;
printf("Contact added.\n");
}
void delete_contact() {
char name[50];
printf("Enter name to delete: ");
scanf("%s", name);
int found = 0;
for (int i = 0; i < num_contacts; i++) {
if (strcmp(contacts[i].name, name) == 0) {
found = 1;
for (int j = i; j < num_contacts - 1; j++) {
contacts[j] = contacts[j + 1];
}
num_contacts--;
printf("Contact deleted.\n");
break;
}
}
if (!found) {
printf("Contact not found.\n");
}
}
void edit_contact() {
char name[50];
printf("Enter name to edit: ");
scanf("%s", name);
int found = 0;
for (int i = 0; i < num_contacts; i++) {
if (strcmp(contacts[i].name, name) == 0) {
found = 1;
printf("Enter new gender: ");
scanf("%s", contacts[i].gender);
printf("Enter new age: ");
scanf("%d", &contacts[i].age);
printf("Enter new home phone: ");
scanf("%s", contacts[i].home_phone);
printf("Enter new mobile phone: ");
scanf("%s", contacts[i].mobile_phone);
printf("Enter new office phone: ");
scanf("%s", contacts[i].office_phone);
printf("Contact edited.\n");
break;
}
}
if (!found) {
printf("Contact not found.\n");
}
}
void search_contact() {
char name[50];
printf("Enter name to search: ");
scanf("%s", name);
int found = 0;
for (int i = 0; i < num_contacts; i++) {
if (strcmp(contacts[i].name, name) == 0) {
found = 1;
printf("Name: %s\n", contacts[i].name);
printf("Gender: %s\n", contacts[i].gender);
printf("Age: %d\n", contacts[i].age);
printf("Home phone: %s\n", contacts[i].home_phone);
printf("Mobile phone: %s\n", contacts[i].mobile_phone);
printf("Office phone: %s\n", contacts[i].office_phone);
break;
}
}
if (!found) {
printf("Contact not found.\n");
}
}
void save_contacts() {
FILE *fp;
fp = fopen("contacts.txt", "w");
if (fp == NULL) {
printf("Error opening file.\n");
return;
}
for (int i = 0; i < num_contacts; i++) {
fprintf(fp, "%s %s %d %s %s %s\n",
contacts[i].name, contacts[i].gender, contacts[i].age,
contacts[i].home_phone, contacts[i].mobile_phone, contacts[i].office_phone);
}
fclose(fp);
printf("Contacts saved to file.\n");
}
void load_contacts() {
FILE *fp;
fp = fopen("contacts.txt", "r");
if (fp == NULL) {
printf("Error opening file.\n");
return;
}
while (!feof(fp) && num_contacts < MAX_CONTACTS) {
struct Contact new_contact;
fscanf(fp, "%s %s %d %s %s %s\n",
new_contact.name, new_contact.gender, &new_contact.age,
new_contact.home_phone, new_contact.mobile_phone, new_contact.office_phone);
contacts[num_contacts++] = new_contact;
}
fclose(fp);
printf("Contacts loaded from file.\n");
}
int main() {
int choice;
while (1) {
printf("\n");
printf("1. Add contact\n");
printf("2. Delete contact\n");
printf("3. Edit contact\n");
printf("4. Search contact\n");
printf("5. Save contacts\n");
printf("6. Load contacts\n");
printf("7. Quit\n");
printf("Enter choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_contact();
break;
case 2:
delete_contact();
break;
case 3:
edit_contact();
break;
case 4:
search_contact();
break;
case 5:
save_contacts();
break;
case 6:
load_contacts();
break;
case 7:
return 0;
default:
printf("Invalid choice.\n");
break;
}
}
}
```
该程序使用结构体 `Contact` 存储联系人信息,其中包含姓名、性别、年龄、家庭电话、移动电话和办公电话。使用结构体数组 `contacts` 存储所有联系人,并使用变量 `num_contacts` 记录当前联系人数量。
程序提供了以下功能:
- 添加联系人:从用户输入中创建一个新的 `Contact` 结构体,并将其添加到 `contacts` 数组中。
- 删除联系人:通过姓名查找联系人,并从 `contacts` 数组中删除它。
- 编辑联系人:通过姓名查找联系人,并允许用户修改其其他信息。
- 查找联系人:通过姓名查找并显示联系人的所有信息。
- 保存联系人:将所有联系人信息写入名为 `contacts.txt` 的文件中。
- 加载联系人:从名为 `contacts.txt` 的文件中读取所有联系人信息,并将其添加到 `contacts` 数组中。
程序使用了菜单循环,用户可以通过输入菜单选项来执行所需的操作。
相关推荐
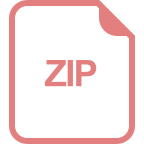
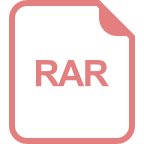














