C++ 定义形状类并派生圆类和矩形类 定义点类为圆类和矩形类定义合适的属性,然后定义成员函数计算面积
时间: 2024-10-10 16:02:11 浏览: 34
在C++中,我们可以创建一个基础形状类,然后通过继承机制派生出更具体的圆类(Circle)和矩形类(Rectangle)。首先,我们定义一个`Shape`类作为基类:
```cpp
class Shape {
protected:
// 点类 Point 的引用
Point center;
public:
// 构造函数,接收点类实例
Shape(Point p) : center(p) {}
// 计算形状面积的基础方法,需由子类重写
virtual double getArea() const = 0;
};
```
接着,我们定义`Point`类:
```cpp
class Point {
public:
double x, y;
Point(double x, double y) : x(x), y(y) {}
};
// 圆类 Circle
class Circle : public Shape {
private:
double radius;
public:
Circle(Point p, double r) : Shape(p), radius(r) {}
// 重写 getArea() 成员函数
double getArea() const override {
return M_PI * pow(radius, 2);
}
};
// 矩形类 Rectangle
class Rectangle : public Shape {
private:
double width, height;
public:
Rectangle(Point p, double w, double h) : Shape(p), width(w), height(h) {}
// 重写 getArea() 成员函数
double getArea() const override {
return width * height;
}
};
```
现在,`Circle`和`Rectangle`类都实现了`getArea()`方法,可以根据它们各自的特性来计算面积。这里`Shape`类的`getArea()`是一个纯虚函数,使得所有从`Shape`派生的类都需要实现它。
阅读全文
相关推荐
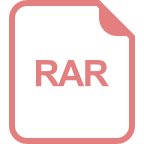
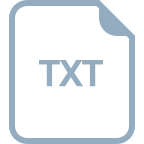



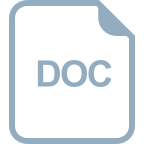
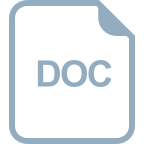
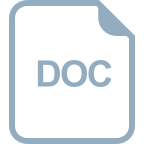








