在 C++ 中,如何设计一个基类形状,以及其派生类圆和矩形,同时让点类成为这两个图形类的共用部分?请具体说明如何为圆和矩形类定义适当的属性,并为它们各自及共享的点类设计计算面积的成员函数。
时间: 2024-10-19 08:12:20 浏览: 28
在C++中,我们可以首先创建一个名为`Shape`的基类,作为所有图形的通用抽象。然后,我们可以为`Circle`和`Rectangle`两个图形派生类添加各自的特有属性,如半径、中心点等,以及共用的点类实例。
```cpp
// 点类 Point
class Point {
public:
double x;
double y;
// 构造函数和必要的运算符重载,例如加法和距离计算
Point(double x = 0, double y = 0) : x(x), y(y) {}
Point operator+(const Point& other) { return {x + other.x, y + other.y}; }
double distance(const Point& other) const { return std::sqrt(std::pow(other.x - x, 2) + std::pow(other.y - y, 2)); }
};
// 基类 Shape
class Shape {
protected:
Point center; // 共享点类的实例
public:
// 计算面积的纯虚函数,每个派生类都需要实现
virtual double area() const = 0;
};
// 派生类 Circle
class Circle : public Shape {
private:
double radius;
public:
Circle(Point center, double radius) : Shape(center), radius(radius) {}
double area() const override { return M_PI * radius * radius; }
};
// 派生类 Rectangle
class Rectangle : public Shape {
private:
double width;
double height;
public:
Rectangle(Point corner, double width, double height) : Shape(corner), width(width), height(height) {}
double area() const override { return width * height; }
};
```
在这个例子中,`Point`类被声明为`Shape`的成员,因此可以被`Circle`和`Rectangle`类的实例访问。每个派生类都重写了`area()`函数,提供自己的面积计算方法。
阅读全文
相关推荐
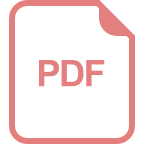
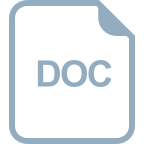
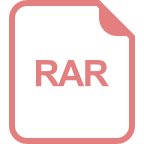















