int binarySearch(vector<int>& nums, int target, bool lower) { int left = 0, right = (int)nums.size() - 1, ans = (int)nums.size(); while (left <= right) { int mid = (left + right) / 2; if (nums[mid] > target || (lower && nums[mid] >= target)) { right = mid - 1; ans = mid; } else { left = mid + 1; } } return ans; } int search(vector<int>& nums, int target) { int leftIdx = binarySearch(nums, target, true); int rightIdx = binarySearch(nums, target, false) - 1; if (leftIdx <= rightIdx && rightIdx < nums.size() && nums[leftIdx] == target && nums[rightIdx] == target) { return rightIdx - leftIdx + 1; } return 0; } 详细解释代码
时间: 2024-01-15 19:03:40 浏览: 150
这段代码实现了在有序数组中查找某个元素的出现次数。
函数 `binarySearch` 是一个二分查找函数,其中 `nums` 是有序数组,`target` 是目标值,`lower` 表示是否查找第一个大于等于目标值的元素。如果 `lower` 为 true,则查找第一个大于等于目标值的元素,否则查找第一个大于目标值的元素。如果找到了符合条件的元素,则返回其下标;否则,返回数组的长度。
函数 `search` 利用 `binarySearch` 函数分别查找目标值在有序数组中第一次和最后一次出现的位置,然后计算出现次数。具体步骤如下:
1. 调用 `binarySearch(nums, target, true)` 查找目标值第一次出现的位置,保存在变量 `leftIdx` 中。
2. 调用 `binarySearch(nums, target, false) - 1` 查找目标值最后一次出现的位置,然后减 1,保存在变量 `rightIdx` 中。
3. 如果目标值在数组中出现过,并且第一次和最后一次出现的位置都找到了,则返回它们之间的元素个数,即 `rightIdx - leftIdx + 1`。
4. 如果目标值在数组中没有出现过,则返回 0。
值得注意的是,这段代码中的二分查找算法是一个通用的模板,可以用来查找大于等于或大于目标值的元素位置。同时,二分查找的时间复杂度为 O(log n),比暴力查找的时间复杂度 O(n) 要快得多。
相关问题
1. 二分查找:在N个无序整数里面查找M个指定整数
好的,针对您的问题,我可以给出以下的二分查找算法实现:
```
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
vector<int> binary_search(vector<int>& nums, vector<int>& targets) {
vector<int> res;
sort(nums.begin(), nums.end());
int n = nums.size();
for (int i = 0; i < targets.size(); ++i) {
int left = 0, right = n - 1;
bool flag = false;
while (left <= right) {
int mid = left + (right - left) / 2;
if (nums[mid] == targets[i]) {
res.push_back(mid);
flag = true;
break;
} else if (nums[mid] < targets[i]) {
left = mid + 1;
} else {
right = mid - 1;
}
}
if (!flag) {
res.push_back(-1);
}
}
return res;
}
int main() {
vector<int> nums = {3, 1, 4, 2, 5};
vector<int> targets = {1, 3, 6};
vector<int> res = binary_search(nums, targets);
for (int i = 0; i < res.size(); ++i) {
cout << res[i] << " ";
}
cout << endl;
return 0;
}
```
上述代码使用了 STL 库中的 sort 函数对原始数组进行排序,时间复杂度为 O(NlogN);然后使用二分查找算法,时间复杂度为 O(MlogN),其中 M 表示指定整数的个数。因此,总时间复杂度为 O((M+N)logN)。
另外,上述代码中的 res 数组中保存了每个指定整数在原始数组中的下标,如果原始数组中不存在对应的指定整数,则返回 -1。
c++ vector查找
C++ 的 vector 类型提供了多种查找元素的方法。下面是一些常用的方法:
1. 使用 find 函数
vector<int> nums = {1, 2, 3, 4, 5};
auto it = find(nums.begin(), nums.end(), 3);
if (it != nums.end()) {
cout << "Found element: " << *it << endl;
} else {
cout << "Element not found" << endl;
}
2. 使用 count 函数
vector<int> nums = {1, 2, 3, 2, 4, 2, 5};
int count = count(nums.begin(), nums.end(), 2);
cout << "Found " << count << " occurrences of 2" << endl;
3. 使用 binary_search 函数
vector<int> nums = {1, 2, 3, 4, 5};
bool found = binary_search(nums.begin(), nums.end(), 3);
if (found) {
cout << "Element found" << endl;
} else {
cout << "Element not found" << endl;
}
注意:在使用这些函数之前,需要包含相应的头文件。例如,find 函数在 algorithm 头文件中定义。
阅读全文
相关推荐
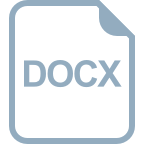
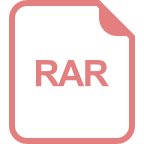
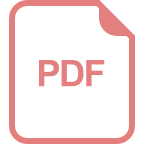












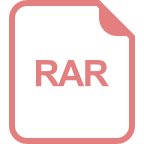
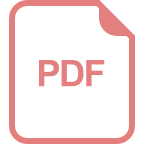