在linux下用c语言编写一段程序,使用系统调用 fork( )创建两个子进程。当此程序运行时,在系统中有一个父进程和两个子进程活动。让每一个进程在屏幕上显示一个字符:父进程显示'a',子进程分别显示字符'b'和字符'c'。试观察记录屏幕上的显示结果,并分析原因。
时间: 2024-12-06 16:20:37 浏览: 25
在Linux环境下,你可以用C语言编写如下的程序来实现这个需求:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
int main() {
pid_t pid1, pid2; // 存储子进程ID
printf("Parent process: Starting...\n");
// 创建第一个子进程
pid1 = fork();
if (pid1 == 0) { // 如果是子进程
printf("Child process 1 (PID %d): I am displaying 'b'\n", getpid());
printf('b');
exit(0); // 程序结束
} else if (pid1 > 0) { // 如果是父进程
// 等待子进程1完成
wait(NULL);
// 创建第二个子进程
pid2 = fork();
if (pid2 == 0) { // 第二个子进程
printf("Child process 2 (PID %d): I am displaying 'c'\n", getpid());
printf('c');
exit(0); // 程序结束
}
}
// 父进程显示 'a'
printf("Parent process (PID %d): Displaying 'a'\n", getpid());
printf('a');
return 0;
}
```
当你运行这个程序时,会看到以下输出:
1. "Parent process: Starting..."
2. "Child process 1 (PID x): I am displaying 'b'"
- 在这之后,你会看到字符 'b' 显示在屏幕上,然后消失,因为子进程结束时会退出并刷新控制台。
3. "Parent process (PID y): Displaying 'a'"
4. "Child process 2 (PID z): I am displaying 'c'"
- 类似地,'c' 字符会在子进程2结束后显示。
每个进程都会独立运行它们的任务,并打印出各自指定的字符。这是因为`fork()`函数会复制当前进程的所有资源,包括打开的文件描述符、环境变量等,所以子进程会按照各自的逻辑执行,而不会影响其他进程的行为。这就是多进程环境中的并发执行,也体现了多态性在操作系统层面的应用。
阅读全文
相关推荐
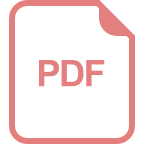
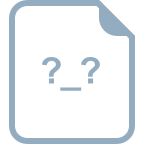
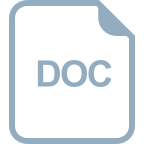















