用c语言 设计一个函数,用于打印右侧显示的图形(“□”表示空格)。该形状具有K线,并且每条线在线前至少有N个空格。每列中的空格数和字母数取决于K。K和N必须从键盘读取。K必须大于0且小于10。准备一个程序来演示如何使用您的函数。
时间: 2024-09-29 21:02:00 浏览: 29
在C语言中,你可以设计一个名为`print_shape`的函数来打印这种特定的图形。这个函数会接收两个整数参数`K`和`N`作为输入,然后按照描述生成并打印出所需的图形。下面是一个简单的函数实现示例:
```c
#include <stdio.h>
#include <string.h>
void print_shape(int K, int N) {
// 验证输入的有效性
if (K <= 0 || K >= 10 || K > N) {
printf("Invalid input! K should be a positive integer less than 10 and greater than N.\n");
return;
}
// 初始化字符串,用于存储每一行的格式
char line[2 * K + 1]; // 包含K个字符(包括'□')和两边的空格
// 生成并填充字符串
for (int i = 0; i <= K; ++i) {
int num_spaces = N - i;
int num_chars = K - i;
// 在左边添加空格
strncpy(line, " ", num_spaces);
// 添加字符 '□'
strcat(line, "[");
// 在右边添加更多空格
strcat(line, " " * num_chars);
// 结尾添加换行符
strcat(line, "\n");
// 输出当前行
printf("%s", line);
}
}
int main() {
int K, N;
printf("Enter the value of K (1-9): ");
scanf("%d", &K);
printf("Enter the minimum number of spaces before each line (K < N): ");
scanf("%d", &N);
print_shape(K, N);
//
阅读全文
相关推荐
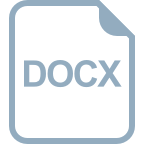
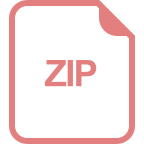
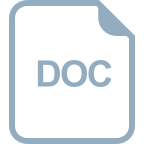




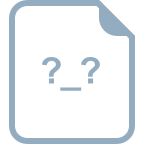
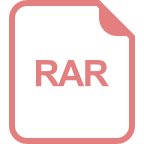
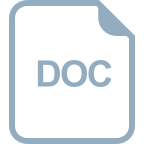
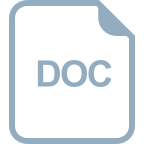
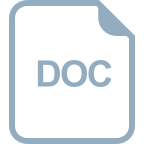





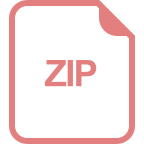