pyqt5 qpoint
时间: 2023-09-07 19:13:55 浏览: 189
QPoint 是 PyQt5 中的一个类,用于表示二维平面上的一个点。它具有以下常用的属性和方法:
属性:
- x:点的 x 坐标
- y:点的 y 坐标
方法:
- QPoint(x, y):创建一个具有指定坐标的 QPoint 对象
- dotProduct(QPoint):计算当前点与给定点的点积
- isNull():检查当前点是否为空点(即坐标为 (0, 0))
- manhattanLength():计算当前点到原点(即 (0, 0))的曼哈顿距离
- setX(int):设置点的 x 坐标
- setY(int):设置点的 y 坐标
- toTuple():将点转换为一个元组 (x, y)
使用 QPoint 可以方便地进行二维平面上的坐标操作和计算。例如,可以使用 QPoint 创建一个点对象,然后通过调用其方法获取或设置其坐标,还可以进行点积、距离计算等操作。
相关问题
PyQt5 localIndexAt
PyQt5 localIndexAt() 方法返回窗口部件的坐标下标。
语法:
```python
localIndexAt(QPoint)
```
参数:
* QPoint:用于指定窗口部件的坐标。
返回值:
* 如果找到该点,则返回该点的局部索引;否则返回-1。
例子:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPlainTextEdit, QVBoxLayout
class Example(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
vbox = QVBoxLayout()
self.textedit = QPlainTextEdit()
self.textedit.setPlainText('Hello\n\nWorld')
vbox.addWidget(self.textedit)
self.setLayout(vbox)
self.setGeometry(300, 300, 300, 150)
self.setWindowTitle('QPlainTextEdit')
self.show()
def mousePressEvent(self, event):
index = self.textedit.localIndexAt(event.pos())
print('localIndex:', index)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
```
在这个例子中,我们创建了一个QPlainTextEdit窗口部件,并在其内部添加了一个文本内容。然后,我们在鼠标按下事件中调用localIndexAt()方法来获取QPlainTextEdit中鼠标点击的局部索引。最后,我们将该索引打印到控制台上。
当我们运行上述代码时,我们可以在QPlainTextEdit中单击鼠标,并在控制台中看到打印出的局部索引。
pyqt5 旋转动画教程
下面是一个简单的 PyQT5 旋转动画示例,可以帮助您入门。该动画将一个圆形图标旋转 360 度,让我们先看一下代码:
```python
from PyQt5.QtCore import Qt, QPropertyAnimation, QPoint
from PyQt5.QtGui import QIcon, QPainter, QPixmap
from PyQt5.QtWidgets import QApplication, QGraphicsWidget, QGraphicsScene, QGraphicsView, QPushButton
class RotatingButton(QPushButton):
def __init__(self, icon, parent=None):
super().__init__(parent)
self.setIcon(icon)
self.setFixedSize(50, 50)
self.setMask(QPixmap(icon).mask())
self.animation = QPropertyAnimation(self, b"pos", self)
self.animation.setDuration(5000)
self.animation.setStartValue(QPoint(100, 100))
self.animation.setEndValue(QPoint(400, 400))
self.animation.setLoopCount(-1)
self.animation.start()
def paintEvent(self, event):
painter = QPainter(self)
painter.translate(self.width() / 2, self.height() / 2)
painter.rotate(self.animation.currentLoopTime() / 16.0)
painter.translate(-self.width() / 2, -self.height() / 2)
super().paintEvent(event)
if __name__ == '__main__':
app = QApplication([])
app.setApplicationName("Rotating Button")
scene = QGraphicsScene()
view = QGraphicsView(scene)
icon = QIcon("icon.png")
button = RotatingButton(icon)
scene.addItem(button)
view.show()
app.exec_()
```
首先,我们定义了一个 `RotatingButton` 类,它继承自 `QPushButton`。构造函数中,我们设置了按钮的图标、大小和遮罩。
接下来,我们创建了一个 `QPropertyAnimation` 实例,并将其与按钮的位置属性相关联。然后,我们设置了动画的持续时间、起始值、结束值和循环次数,并开始运行动画。
在 `paintEvent` 函数中,我们首先创建了一个 `QPainter` 实例,并将其平移至按钮中心。然后,我们根据当前循环时间旋转了画布,并再次平移回按钮左上角。最后,我们调用了 `super().paintEvent(event)` 来绘制按钮的图标。
最后,在 `if __name__ == '__main__':` 块中,我们创建了一个 `QGraphicsScene` 和一个 `QGraphicsView` 实例。我们使用 `RotatingButton` 类创建了一个旋转的按钮,并将其添加到场景中。最后,我们显示了视图并开始 PyQT5 应用程序的事件循环。
这就是一个简单的 PyQT5 旋转动画示例,希望能对您有所帮助!
阅读全文
相关推荐
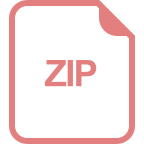
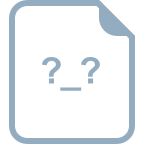
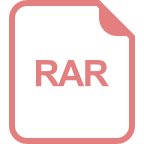
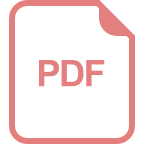
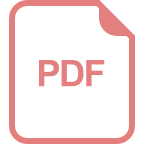
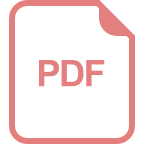









