用c++实现输入两个稀疏一元多项式表达式,然后对两个表达式求解
时间: 2023-12-14 13:39:04 浏览: 194
这里提供一种基于链表的稀疏多项式表达式求解方法。
首先,我们需要定义一个结构体来表示多项式中的每一项:
```c++
struct PolyTerm {
int coefficient; // 系数
int exponent; // 指数
PolyTerm* next; // 下一项
};
```
然后,我们可以定义一个多项式类,其中包含两个成员变量 `head` 和 `tail` 分别表示多项式的头结点和尾结点。同时,我们也需要定义一些方法来对多项式进行操作,如插入项、合并同类项等。
```c++
class SparsePolynomial {
public:
SparsePolynomial() {
head = tail = nullptr;
}
// 插入项
void insert(int coefficient, int exponent) {
if (coefficient == 0) return; // 如果系数为0,则不进行插入
PolyTerm* newTerm = new PolyTerm{coefficient, exponent, nullptr};
if (head == nullptr) { // 如果多项式为空,则新插入的项为头结点
head = tail = newTerm;
} else { // 否则插入到尾结点后面
tail->next = newTerm;
tail = newTerm;
}
}
// 合并同类项
void merge() {
PolyTerm* p = head;
while (p != nullptr) {
PolyTerm* q = p->next;
while (q != nullptr && q->exponent == p->exponent) { // 如果指数相同,则合并同类项
p->coefficient += q->coefficient;
PolyTerm* tmp = q;
q = q->next;
delete tmp;
}
p->next = q;
p = q;
}
}
// 打印多项式
void print() {
PolyTerm* p = head;
while (p != nullptr) {
cout << p->coefficient << "x^" << p->exponent;
p = p->next;
if (p != nullptr) {
cout << " + ";
}
}
cout << endl;
}
private:
PolyTerm* head;
PolyTerm* tail;
};
```
接下来,我们可以编写主函数,首先输入两个多项式,然后将它们合并同类项并打印结果。
```c++
int main() {
SparsePolynomial poly1, poly2;
int n1, n2;
cout << "输入多项式1的项数: ";
cin >> n1;
for (int i = 0; i < n1; i++) {
int coefficient, exponent;
cout << "输入第" << i + 1 << "项的系数和指数: ";
cin >> coefficient >> exponent;
poly1.insert(coefficient, exponent);
}
cout << "输入多项式2的项数: ";
cin >> n2;
for (int i = 0; i < n2; i++) {
int coefficient, exponent;
cout << "输入第" << i + 1 << "项的系数和指数: ";
cin >> coefficient >> exponent;
poly2.insert(coefficient, exponent);
}
poly1.merge();
poly2.merge();
cout << "多项式1: ";
poly1.print();
cout << "多项式2: ";
poly2.print();
return 0;
}
```
当然,这只是一个基本的实现,还可以对其中的一些方法进行优化,例如插入项时可以按指数大小排序,合并同类项时可以使用哈希表等数据结构来提高效率。
阅读全文
相关推荐
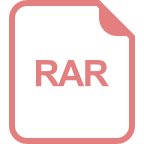
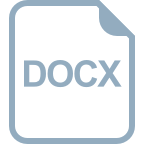


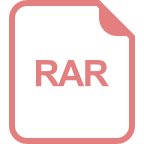
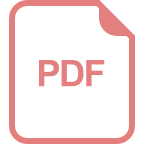
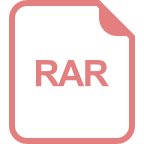
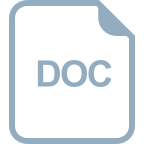
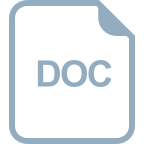
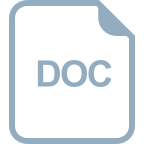
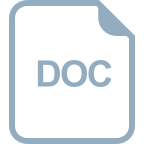
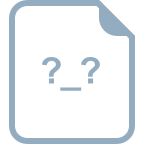