用Java写一个原型设计模式的深拷贝
时间: 2023-05-21 12:01:41 浏览: 122
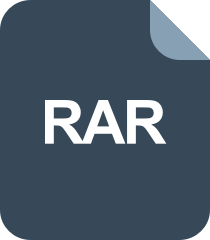
java设计模式【之】原型模式、深拷贝与浅拷贝【源码】【场景:克隆羊】

可以使用Java中的序列化来实现原型设计模式的深拷贝。具体实现步骤如下:
1. 创建一个需要进行深拷贝的类,并实现Serializable接口。
2. 在该类中添加一个方法,用于将该类序列化为字节数组。
3. 在该类中添加一个静态方法,用于将字节数组反序列化为该类的实例。
4. 在需要进行深拷贝的地方,调用序列化方法将该对象序列化为字节数组。
5. 调用反序列化方法将字节数组反序列化为新的对象,即可完成深拷贝。
以下是一个示例代码:
import java.io.*;
public class Prototype implements Serializable {
private String name;
private int age;
public Prototype(String name, int age) {
this.name = name;
this.age = age;
}
public byte[] serialize() throws IOException {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(bos);
oos.writeObject(this);
return bos.toByteArray();
}
public static Prototype deserialize(byte[] data) throws IOException, ClassNotFoundException {
ByteArrayInputStream bis = new ByteArrayInputStream(data);
ObjectInputStream ois = new ObjectInputStream(bis);
return (Prototype) ois.readObject();
}
}
在需要进行深拷贝的地方,可以这样使用:
Prototype p1 = new Prototype("Tom", 20);
byte[] data = p1.serialize();
Prototype p2 = Prototype.deserialize(data);
此时,p2就是p1的深拷贝。
阅读全文
相关推荐

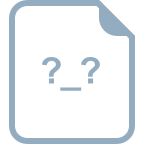
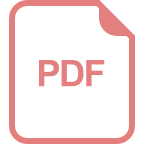
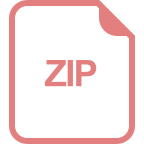
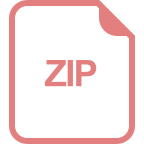
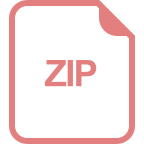
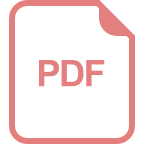
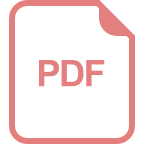
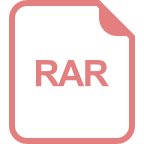
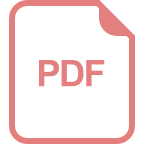
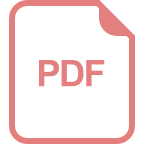
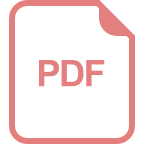
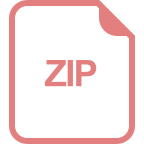
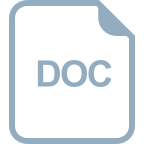
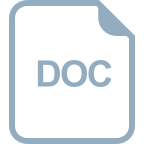
