在Python中怎么实现Dijkstra算法:其中比较每个初始点终点的最短路径
时间: 2024-02-28 20:56:37 浏览: 23
实现Dijkstra算法的一种常见方式是使用优先级队列来维护图中每个节点的距离值,算法的基本思路是:
1. 初始化距离值,将起点的距离值设为0,其他节点距离值设为无穷大。
2. 将起点加入优先级队列中。
3. 取出队列中距离值最小的节点,遍历该节点的所有邻居节点。
4. 对于每个邻居节点,计算从起点到该节点的距离值,如果该距离值小于该节点当前的距离值,则更新该节点的距离值,并将该节点加入队列中。
5. 重复步骤3和4,直到队列为空或者找到终点。
以下是一个简单的Python实现:
```python
import heapq
def dijkstra(graph, start, end):
distances = {node: float('inf') for node in graph}
distances[start] = 0
queue = [(0, start)]
while queue:
current_distance, current_node = heapq.heappop(queue)
if current_node == end:
return distances[end]
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(queue, (distance, neighbor))
return float('inf')
```
其中,`graph`是图的邻接表表示,`start`和`end`分别是起点和终点。函数返回起点到终点的最短距离。
相关推荐
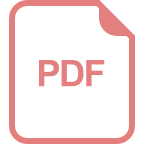
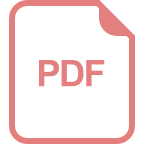
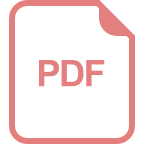














