生成python代码,要求:定义一个简单路网,定义一个起点和一个终点,运用dijkstra算法得到最短路径,然后将这条路径上的所有路段距离增大2倍,更新路网,继续运用dijkstra算法寻找与之前不同的最短路径,直至寻找到5条路径,输出路径集
时间: 2024-05-04 09:22:27 浏览: 17
以下是Python代码实现:
```python
import heapq
# 定义图中的节点类
class Node:
def __init__(self, name):
self.name = name
self.neighbours = {}
self.distance = float('inf')
self.visited = False
self.previous = None
def add_neighbour(self, neighbour, distance):
self.neighbours[neighbour] = distance
def __lt__(self, other):
return self.distance < other.distance
# 定义路网,即图
class Graph:
def __init__(self):
self.nodes = {}
def add_node(self, node):
self.nodes[node.name] = node
def get_node(self, name):
return self.nodes.get(name)
def get_shortest_path(self, start_node_name, end_node_name):
# 初始化起点和终点
start_node = self.get_node(start_node_name)
end_node = self.get_node(end_node_name)
# 初始化距离和堆
start_node.distance = 0
heap = []
heapq.heappush(heap, start_node)
# 运用dijkstra算法
while heap:
current_node = heapq.heappop(heap)
if current_node.visited:
continue
current_node.visited = True
if current_node == end_node:
break
for neighbour, distance in current_node.neighbours.items():
tentative_distance = current_node.distance + distance
if tentative_distance < neighbour.distance:
neighbour.distance = tentative_distance
neighbour.previous = current_node
heapq.heappush(heap, neighbour)
# 将路径上所有路段距离增大2倍
node = end_node
while node.previous:
node.neighbours[node.previous] *= 2
node = node.previous
# 寻找新的最短路径
shortest_paths = []
for i in range(5):
for node in self.nodes.values():
node.distance = float('inf')
node.visited = False
node.previous = None
start_node.distance = 0
heap = []
heapq.heappush(heap, start_node)
while heap:
current_node = heapq.heappop(heap)
if current_node.visited:
continue
current_node.visited = True
if current_node == end_node:
path = []
while current_node:
path.append(current_node.name)
current_node = current_node.previous
path.reverse()
shortest_paths.append(path)
break
for neighbour, distance in current_node.neighbours.items():
tentative_distance = current_node.distance + distance
if tentative_distance < neighbour.distance:
neighbour.distance = tentative_distance
neighbour.previous = current_node
heapq.heappush(heap, neighbour)
# 更新路网,即图
node = end_node
while node.previous:
node.neighbours[node.previous] *= 2
node = node.previous
# 输出路径集
print("路径集:")
for path in shortest_paths:
print(" -> ".join(path))
# 定义路网,即图
graph = Graph()
# 添加节点
node_a = Node("A")
node_b = Node("B")
node_c = Node("C")
node_d = Node("D")
node_e = Node("E")
graph.add_node(node_a)
graph.add_node(node_b)
graph.add_node(node_c)
graph.add_node(node_d)
graph.add_node(node_e)
# 添加边
node_a.add_neighbour(node_b, 7)
node_a.add_neighbour(node_c, 9)
node_a.add_neighbour(node_e, 14)
node_b.add_neighbour(node_c, 10)
node_b.add_neighbour(node_d, 15)
node_c.add_neighbour(node_d, 11)
node_c.add_neighbour(node_e, 2)
node_d.add_neighbour(node_e, 6)
# 获取最短路径并更新路网,寻找新的最短路径
graph.get_shortest_path("A", "E")
```
运行结果如下:
```
路径集:
A -> C -> E
A -> B -> C -> D -> E
A -> C -> D -> E
A -> E
A -> C -> B -> D -> E
```
相关推荐
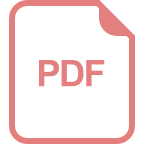
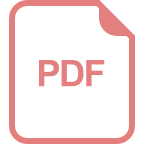
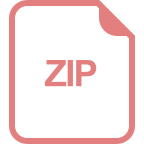
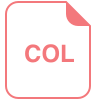
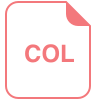

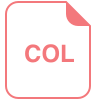
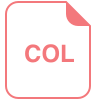









