请用java语言写一个贪吃蛇
时间: 2023-05-20 21:05:30 浏览: 52
好的,以下是一个简单的贪吃蛇游戏的Java代码:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class SnakeGame extends JFrame implements ActionListener {
private Timer timer;
private Snake snake;
private Food food;
private boolean gameOver;
public SnakeGame() {
setTitle("Snake Game");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
snake = new Snake();
food = new Food(snake);
gameOver = false;
addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if (key == KeyEvent.VK_LEFT) {
snake.setDirection(Snake.Direction.LEFT);
} else if (key == KeyEvent.VK_RIGHT) {
snake.setDirection(Snake.Direction.RIGHT);
} else if (key == KeyEvent.VK_UP) {
snake.setDirection(Snake.Direction.UP);
} else if (key == KeyEvent.VK_DOWN) {
snake.setDirection(Snake.Direction.DOWN);
}
}
});
timer = new Timer(100, this);
timer.start();
pack();
setLocationRelativeTo(null);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (!gameOver) {
snake.move();
if (snake.collidesWith(food)) {
snake.grow();
food = new Food(snake);
}
if (snake.collidesWithItself()) {
gameOver = true;
}
repaint();
}
}
public void paint(Graphics g) {
super.paint(g);
snake.draw(g);
food.draw(g);
if (gameOver) {
g.drawString("Game Over", 150, 150);
}
}
public static void main(String[] args) {
new SnakeGame();
}
}
class Snake {
public enum Direction { LEFT, RIGHT, UP, DOWN };
private static final int BLOCK_SIZE = 10;
private static final int INITIAL_SIZE = 5;
private Direction direction;
private int x[];
private int y[];
private int size;
public Snake() {
direction = Direction.RIGHT;
x = new int[INITIAL_SIZE];
y = new int[INITIAL_SIZE];
size = INITIAL_SIZE;
for (int i = 0; i < size; i++) {
x[i] = BLOCK_SIZE * (size - i);
y[i] = BLOCK_SIZE;
}
}
public void setDirection(Direction d) {
if ((direction == Direction.LEFT && d != Direction.RIGHT) ||
(direction == Direction.RIGHT && d != Direction.LEFT) ||
(direction == Direction.UP && d != Direction.DOWN) ||
(direction == Direction.DOWN && d != Direction.UP)) {
direction = d;
}
}
public void move() {
for (int i = size - 1; i > 0; i--) {
x[i] = x[i - 1];
y[i] = y[i - 1];
}
if (direction == Direction.LEFT) {
x[0] -= BLOCK_SIZE;
} else if (direction == Direction.RIGHT) {
x[0] += BLOCK_SIZE;
} else if (direction == Direction.UP) {
y[0] -= BLOCK_SIZE;
} else if (direction == Direction.DOWN) {
y[0] += BLOCK_SIZE;
}
}
public void grow() {
size++;
int[] newX = new int[size];
int[] newY = new int[size];
for (int i = 0; i < size - 1; i++) {
newX[i] = x[i];
newY[i] = y[i];
}
newX[size - 1] = x[size - 2];
newY[size - 1] = y[size - 2];
x = newX;
y = newY;
}
public boolean collidesWith(Food food) {
return x[0] == food.getX() && y[0] == food.getY();
}
public boolean collidesWithItself() {
for (int i = 1; i < size; i++) {
if (x[0] == x[i] && y[0] == y[i]) {
return true;
}
}
return false;
}
public void draw(Graphics g) {
g.setColor(Color.GREEN);
for (int i = 0; i < size; i++) {
g.fillRect(x[i], y[i], BLOCK_SIZE, BLOCK_SIZE);
}
}
}
class Food {
private static final int BLOCK_SIZE = 10;
private int x;
private int y;
public Food(Snake snake) {
do {
x = (int) (Math.random() * SnakeGame.WIDTH / BLOCK_SIZE) * BLOCK_SIZE;
y = (int) (Math.random() * SnakeGame.HEIGHT / BLOCK_SIZE) * BLOCK_SIZE;
} while (snake.collidesWithItself() || collidesWithSnake(snake));
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public boolean collidesWithSnake(Snake snake) {
for (int i = 0; i < snake.size; i++) {
if (x == snake.x[i] && y == snake.y[i]) {
return true;
}
}
return false;
}
public void draw(Graphics g) {
g.setColor(Color.RED);
g.fillRect(x, y, BLOCK_SIZE, BLOCK_SIZE);
}
}
```
希望这个代码能够帮到你!
相关推荐
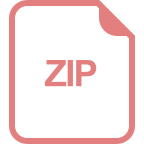
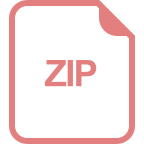














