编写dbscan的代码
时间: 2023-08-31 17:35:00 浏览: 74
### 回答1:
我可以提供一些参考代码,但是在实际应用中,您可能需要根据自己的具体需求对代码进行调整。例如:from sklearn.cluster import DBSCAN
import numpy as np# 设置参数
db = DBSCAN(eps=0.3, min_samples=10).fit(X)
# 找到聚类簇
labels = db.labels_
# 分离聚类簇
clusters = np.unique(labels)
# 计算每个簇的数量
for cluster in clusters:
print('簇:{},数量:{}'.format(cluster, list(labels).count(cluster)))
### 回答2:
DBSCAN(Density-Based Spatial Clustering of Applications with Noise)是一种基于密度的聚类算法,用于将数据集中的样本点按照密度分布进行聚类,并能够识别出离群点。下面是一个简单的DBSCAN算法的代码:
1. 导入相关的库
```python
import numpy as np
import pandas as pd
from sklearn.neighbors import NearestNeighbors
```
2. 定义DBSCAN类
```python
class DBSCAN:
def __init__(self, eps, min_samples):
self.eps = eps
self.min_samples = min_samples
def fit(self, X):
self.X = X
self.labels = [-1] * len(X)
self.cluster_label = 0
for i in range(len(X)):
if self.labels[i] == -1:
if self.expand_cluster(i, self.cluster_label):
self.cluster_label += 1
def expand_cluster(self, core_sample_index, cluster_label):
seeds = self.region_query(core_sample_index)
if len(seeds) < self.min_samples:
self.labels[core_sample_index] = -1
return False
self.labels[core_sample_index] = cluster_label
while seeds:
current_point = seeds.pop(0)
if self.labels[current_point] == -1:
self.labels[current_point] = cluster_label
new_seeds = self.region_query(current_point)
if len(new_seeds) >= self.min_samples:
seeds += new_seeds
elif self.labels[current_point] == 0:
self.labels[current_point] = cluster_label
return True
def region_query(self, point_index):
return [i for i, x in enumerate(self.X) if np.linalg.norm(x - self.X[point_index]) <= self.eps]
```
3. 初始化数据集并执行聚类
```python
data = pd.read_csv("data.csv") # 读取数据集文件
X = data.values # 转换为numpy数组
dbscan = DBSCAN(eps=0.5, min_samples=5)
dbscan.fit(X)
print(dbscan.labels) # 打印样本点的聚类标签
```
在代码中,我们首先导入了必要的库,包括numpy、pandas和sklearn.neighbors.NearestNeighbors。然后定义了一个DBSCAN类,包含了fit、expand_cluster和region_query等方法,用于执行聚类步骤。最后,我们初始化了一个数据集,并调用DBSCAN类的fit方法进行聚类,并输出样本点的聚类标签。
以上是一个简单的DBSCAN算法的代码示例,其中的参数和细节可根据具体需求进行调整和优化。
### 回答3:
DBSCAN(Density-Based Spatial Clustering of Applications with Noise)是一种基于密度的聚类算法,用于发现具有相似密度的数据点集合。下面是一个用Python编写的DBSCAN算法的简单示例代码:
```
import numpy as np
def dbscan(data, epsilon, min_samples):
# 初始化所有点的标识,未分类为0,噪声点为-1
labels = [0] * len(data)
cluster_id = 0
# 对每个点进行遍历
for idx, point in enumerate(data):
# 如果该点已经被分类过,则跳过
if labels[idx] != 0:
continue
# 找到该点的邻居点集合
neighbors = find_neighbors(data, point, epsilon)
# 如果邻居点数量小于min_samples,标记为噪声点
if len(neighbors) < min_samples:
labels[idx] = -1
else:
cluster_id += 1
labels[idx] = cluster_id
expand_cluster(data, labels, point, neighbors, cluster_id, epsilon, min_samples)
return labels
def expand_cluster(data, labels, point, neighbors, cluster_id, epsilon, min_samples):
# 将当前点添加到聚类中
for neighbor in neighbors:
neighbor_index = data.index(neighbor)
if labels[neighbor_index] == 0:
labels[neighbor_index] = cluster_id
# 如果该点还有足够的邻居点,则继续扩展聚类
neighbor_neighbors = find_neighbors(data, neighbor, epsilon)
if len(neighbor_neighbors) >= min_samples:
expand_cluster(data, labels, neighbor, neighbor_neighbors, cluster_id, epsilon, min_samples)
def find_neighbors(data, point, epsilon):
neighbors = []
for neighbor in data:
if np.linalg.norm(point - neighbor) <= epsilon:
neighbors.append(neighbor)
return neighbors
# 使用示例
data = np.array([[1, 2], [2, 1], [2, 3], [8, 7], [8, 9], [7, 8], [25, 80]])
epsilon = 3
min_samples = 2
labels = dbscan(data, epsilon, min_samples)
print(labels)
```
这段代码实现了基本的DBSCAN算法,输入参数包括数据集`data`、邻域半径`epsilon`和最小邻居点数`min_samples`。输出结果为每个数据点的标签,-1表示噪声点,大于0的整数表示聚类id。
相关推荐
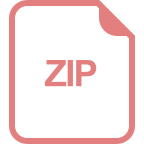
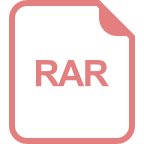
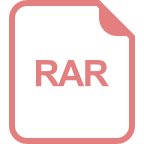














