from mpl_toolkits.mplot3d import Axes3D import matplotlib.pyplot as plt import numpy as np # 生成随机三维数据点 np.random.seed(0) points = np.random.rand(8, 3) # 计算Convex Hull hull = ConvexHull(points) # 可视化Convex Hull fig = plt.figure() ax = fig.add_subplot(111, projection="3d") for s in hull.simplices: ax.plot(points[s, 0], points[s, 2], points[s, 1], "b-") plt.show() from scipy.spatial import distance def douglas_peucker(points, tolerance): """ 使用Douglas-Peucker算法将多边形折线进行简化 :param points: 多边形点集,numpy数组,每行表示一个三维点 :param tolerance: 简化误差容忍度 :return: 简化后的多边形点集 """ if len(points) < 3: return points # 找到距离最远的点作为分割点 dmax = 0 index = 0 end = len(points) - 1 for i in range(1, end): d = distance.point_line_distance(points[i], points[0], points[end]) if d > dmax: index = i dmax = d # 如果距离最远点小于容忍度,则直接返回两个端点 if dmax < tolerance: return np.array([points[0], points[end]]) # 递归地对两个子区间进行简化 left_points = douglas_peucker(points[:index+1], tolerance) right_points = douglas_peucker(points[index:], tolerance) # 返回合并后的结果 return np.vstack((left_points[:-1], right_points)) 请将这两个代码段合并,使其能够接连工作。
时间: 2024-01-28 14:02:58 浏览: 148
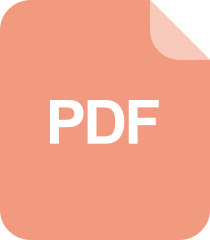
Matplotlib.pyplot 三维绘图的实现示例
代码段一是使用matplotlib库可视化一个三维Convex Hull,代码段二是使用Douglas-Peucker算法对点集进行多边形折线简化。这两段代码需要的依赖库是numpy、matplotlib和scipy.spatial中的distance模块。
合并这两段代码需要在代码段一中添加导入ConvexHull类的代码,并将ConvexHull(points)替换为douglas_peucker(points, tolerance)。
合并后的代码如下:
```python
import numpy as np
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
from scipy.spatial import distance
def douglas_peucker(points, tolerance):
"""
使用Douglas-Peucker算法将多边形折线进行简化
:param points: 多边形点集,numpy数组,每行表示一个三维点
:param tolerance: 简化误差容忍度
:return: 简化后的多边形点集
"""
if len(points) < 3:
return points
# 找到距离最远的点作为分割点
dmax = 0
index = 0
end = len(points) - 1
for i in range(1, end):
d = distance.point_line_distance(points[i], points[0], points[end])
if d > dmax:
index = i
dmax = d
# 如果距离最远点小于容忍度,则直接返回两个端点
if dmax < tolerance:
return np.array([points[0], points[end]])
# 递归地对两个子区间进行简化
left_points = douglas_peucker(points[:index+1], tolerance)
right_points = douglas_peucker(points[index:], tolerance)
# 返回合并后的结果
return np.vstack((left_points[:-1], right_points))
# 生成随机三维数据点
np.random.seed(0)
points = np.random.rand(8, 3)
# 计算多边形折线简化后的点集
tolerance = 0.1
simplified_points = douglas_peucker(points, tolerance)
# 计算Convex Hull
hull = ConvexHull(simplified_points)
# 可视化Convex Hull
fig = plt.figure()
ax = fig.add_subplot(111, projection="3d")
for s in hull.simplices:
ax.plot(simplified_points[s, 0], simplified_points[s, 2], simplified_points[s, 1], "b-")
plt.show()
```
这段代码的运行结果是生成一个简化后的Convex Hull的三维可视化图形。
阅读全文
相关推荐
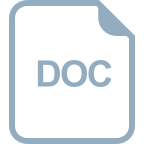
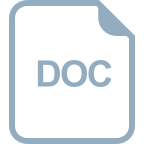














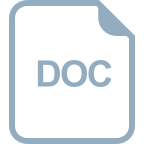
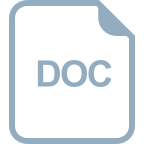