ffmpeg+sdl2实现带音量柱的播放器
时间: 2023-11-10 08:06:28 浏览: 247
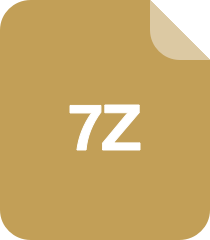
FFmpeg/SDL2实现音视频播放库
你可以使用FFmpeg和SDL2库来实现带有音量柱的播放器。下面是一个简单的示例代码,可以帮助你开始:
```c
#include <stdio.h>
#include <SDL2/SDL.h>
#include <libavformat/avformat.h>
#include <libswresample/swresample.h>
#define SAMPLE_RATE 44100
#define CHANNELS 2
#define BUFFER_SIZE 4096
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("Usage: %s <input_file>\n", argv[0]);
return -1;
}
const char *inputFile = argv[1];
// 初始化SDL
if (SDL_Init(SDL_INIT_AUDIO) < 0) {
printf("SDL initialization failed: %s\n", SDL_GetError());
return -1;
}
// 打开输入文件
AVFormatContext *formatContext = NULL;
if (avformat_open_input(&formatContext, inputFile, NULL, NULL) != 0) {
printf("Failed to open input file\n");
return -1;
}
// 查找音频流信息
if (avformat_find_stream_info(formatContext, NULL) < 0) {
printf("Failed to find stream information\n");
return -1;
}
// 查找音频流索引
int audioStreamIndex = -1;
for (int i = 0; i < formatContext->nb_streams; i++) {
if (formatContext->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_AUDIO) {
audioStreamIndex = i;
break;
}
}
if (audioStreamIndex == -1) {
printf("Failed to find audio stream\n");
return -1;
}
// 获取音频流解码参数
AVCodecParameters *codecParams = formatContext->streams[audioStreamIndex]->codecpar;
// 查找音频解码器
AVCodec *codec = avcodec_find_decoder(codecParams->codec_id);
if (codec == NULL) {
printf("Failed to find decoder\n");
return -1;
}
// 创建解码器上下文
AVCodecContext *codecContext = avcodec_alloc_context3(codec);
if (avcodec_parameters_to_context(codecContext, codecParams) != 0) {
printf("Failed to copy decoder parameters to context\n");
return -1;
}
// 打开解码器
if (avcodec_open2(codecContext, codec, NULL) != 0) {
printf("Failed to open decoder\n");
return -1;
}
// 创建音频重采样上下文
SwrContext *swrContext = swr_alloc();
av_opt_set_int(swrContext, "in_channel_layout", codecContext->channel_layout, 0);
av_opt_set_int(swrContext, "out_channel_layout", codecContext->channel_layout, 0);
av_opt_set_int(swrContext, "in_sample_rate", codecContext->sample_rate, 0);
av_opt_set_int(swrContext, "out_sample_rate", SAMPLE_RATE, 0);
av_opt_set_sample_fmt(swrContext, "in_sample_fmt", codecContext->sample_fmt, 0);
av_opt_set_sample_fmt(swrContext, "out_sample_fmt", AV_SAMPLE_FMT_S16, 0);
swr_init(swrContext);
// 设置SDL音频参数
SDL_AudioSpec audioSpec;
audioSpec.freq = SAMPLE_RATE;
audioSpec.format = AUDIO_S16SYS;
audioSpec.channels = CHANNELS;
audioSpec.silence = 0;
audioSpec.samples = BUFFER_SIZE;
audioSpec.callback = NULL; // TODO: 实现音频回调函数
audioSpec.userdata = codecContext;
// 打开音频设备
if (SDL_OpenAudio(&audioSpec, NULL) < 0) {
printf("Failed to open audio device: %s\n", SDL_GetError());
return -1;
}
// 开始播放
SDL_PauseAudio(0);
// 解码并播放音频数据
AVPacket packet;
av_init_packet(&packet);
AVFrame *frame = av_frame_alloc();
uint8_t *buffer = (uint8_t *) av_malloc(MAX_AUDIO_FRAME_SIZE);
while (av_read_frame(formatContext, &packet) >= 0) {
if (packet.stream_index == audioStreamIndex) {
int ret = avcodec_send_packet(codecContext, &packet);
if (ret < 0) {
printf("Error sending a packet for decoding\n");
break;
}
while (ret >= 0) {
ret = avcodec_receive_frame(codecContext, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
else if (ret < 0) {
printf("Error during decoding\n");
break;
}
// 音频重采样
int dstNbSamples = av_rescale_rnd(swr_get_delay(swrContext, frame->sample_rate) + frame->nb_samples,
SAMPLE_RATE, frame->sample_rate, AV_ROUND_UP);
int nb = swr_convert(swrContext, &buffer, dstNbSamples, (const uint8_t **) frame->data, frame->nb_samples);
int audioSize = nb * CHANNELS * av_get_bytes_per_sample(AV_SAMPLE_FMT_S16);
// TODO: 更新音量柱
// 播放音频数据
SDL_QueueAudio(1, buffer, audioSize);
}
}
av_packet_unref(&packet);
}
// 清理资源
av_frame_free(&frame);
av_free(buffer);
swr_free(&swrContext);
avcodec_close(codecContext);
avformat_close_input(&formatContext);
SDL_CloseAudio();
SDL_Quit();
return 0;
}
```
这只是一个简单的示例代码,你可能需要根据你的需求进行修改和扩展。要实现音量柱功能,你可以在音频回调函数中计算音频样本的能量,并将其映射到柱状图上。希望这对你有所帮助!
阅读全文
相关推荐
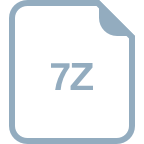
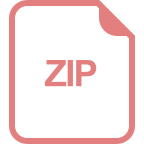
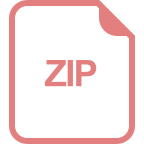
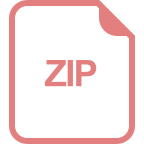
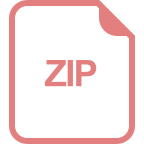
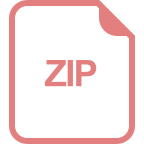
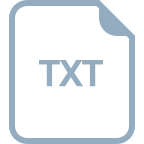