用c语言利用栈和队列实现输入10进制数将它转换成8进制数
时间: 2024-03-17 20:43:38 浏览: 69
好的,我来回答你的问题。
首先,我们可以定义一个栈和一个队列来实现这个功能。
栈用来存储转换后的8进制数,队列用来存储10进制数每一位的余数。
具体实现步骤如下:
1. 定义一个空的栈和队列。
2. 将输入的10进制数不断除以8,将每一次的余数放入队列中,直到商为0。
3. 从队列中取出每一个余数,依次放入栈中。
4. 依次从栈中取出每一个余数,拼接成8进制数的字符串。
5. 输出转换后的8进制数。
下面是代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
// 定义栈结构体
typedef struct {
int data[MAX_SIZE];
int top;
} Stack;
// 初始化栈
void init_stack(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int is_empty(Stack *s) {
return s->top == -1;
}
// 判断栈是否已满
int is_full(Stack *s) {
return s->top == MAX_SIZE - 1;
}
// 入栈
void push(Stack *s, int x) {
if (is_full(s)) {
printf("Stack Overflow!\n");
exit(1);
}
s->data[++s->top] = x;
}
// 出栈
int pop(Stack *s) {
if (is_empty(s)) {
printf("Stack Underflow!\n");
exit(1);
}
return s->data[s->top--];
}
// 定义队列结构体
typedef struct {
int data[MAX_SIZE];
int front;
int rear;
} Queue;
// 初始化队列
void init_queue(Queue *q) {
q->front = q->rear = -1;
}
// 判断队列是否为空
int is_queue_empty(Queue *q) {
return q->front == -1;
}
// 判断队列是否已满
int is_queue_full(Queue *q) {
return q->front == (q->rear + 1) % MAX_SIZE;
}
// 入队
void enqueue(Queue *q, int x) {
if (is_queue_full(q)) {
printf("Queue Overflow!\n");
exit(1);
}
if (is_queue_empty(q)) {
q->front = q->rear = 0;
} else {
q->rear = (q->rear + 1) % MAX_SIZE;
}
q->data[q->rear] = x;
}
// 出队
int dequeue(Queue *q) {
if (is_queue_empty(q)) {
printf("Queue Underflow!\n");
exit(1);
}
int x = q->data[q->front];
if (q->front == q->rear) {
q->front = q->rear = -1;
} else {
q->front = (q->front + 1) % MAX_SIZE;
}
return x;
}
// 将10进制数转换成8进制数
void convert_to_octal(int n) {
Stack s;
Queue q;
init_stack(&s);
init_queue(&q);
while (n > 0) {
int remainder = n % 8;
enqueue(&q, remainder);
n /= 8;
}
while (!is_queue_empty(&q)) {
int remainder = dequeue(&q);
push(&s, remainder);
}
printf("The octal number is: ");
while (!is_empty(&s)) {
printf("%d", pop(&s));
}
printf("\n");
}
int main() {
int n;
printf("Please enter a decimal number: ");
scanf("%d", &n);
convert_to_octal(n);
return 0;
}
```
希望这个程序能够帮助到你!
阅读全文
相关推荐






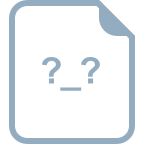
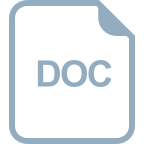
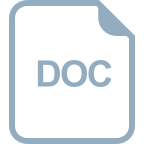
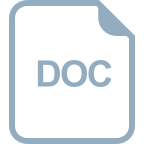
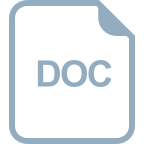
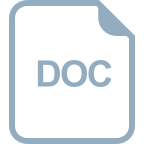
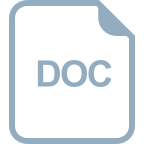
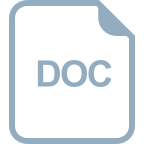
