c语言中栈和队列写编写主函数来调用上述函数体,设计并实现算法:十进制转换为二进制 简易版
时间: 2024-10-25 15:06:51 浏览: 38
在C语言中,我们可以使用数组模拟栈和队列来实现简单的十进制转二进制算法。这里我们只做基本的整数转换,不涉及更复杂的浮点数或字符串处理。下面是一个简化的十进制转二进制的示例,使用栈来存储二进制位:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
// Stack for binary digits
#define STACK_SIZE 10
typedef struct {
int stack[STACK_SIZE];
size_t top;
} Stack;
// Push an element onto the stack
void push(Stack *s, int digit) {
if (s->top == STACK_SIZE) {
printf("Stack overflow!\n");
return;
}
s->stack[s->top++] = digit;
}
// Pop an element from the stack
int pop(Stack *s) {
if (s->top == 0) {
printf("Stack underflow!\n");
return -1; // Return a sentinel value to indicate empty stack
}
return s->stack[--s->top];
}
// Convert decimal to binary using recursion
bool decimalToBinary(int num, Stack *s) {
if (num > 0) {
push(s, num % 2);
decimalToBinary(num / 2, s);
} else if (num == 0) {
return true;
}
return false;
}
int main() {
int decimal_num;
printf("Enter a decimal number: ");
scanf("%d", &decimal_num);
Stack binary_stack;
binary_stack.top = 0;
if (!decimalToBinary(decimal_num, &binary_stack)) {
while (binary_stack.top != 0) {
int digit = pop(&binary_stack);
printf("%d", digit); // Output the binary digits in reverse order
}
printf("\n");
}
return 0;
}
```
在这个程序中,用户输入一个十进制数,然后通过递归将它转换为二进制,并把结果存入栈中。最后从栈中弹出数字,得到的就是二进制表示。
阅读全文
相关推荐




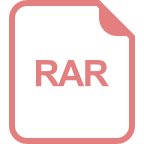

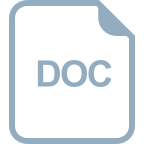
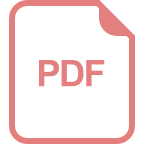









