bwriter.Write(this.IntToByte((uint)r.station.stationId,1));
时间: 2024-02-25 17:59:29 浏览: 37
这段代码是将r.station.stationId转换为1字节的无符号整数,并写入到二进制流中。具体实现是调用了IntToByte方法将r.station.stationId转换为字节数组,并使用BinaryWriter类的Write方法将字节数组写入到二进制流中。IntToByte方法的实现可能类似于以下代码:
```
public byte[] IntToByte(uint value, int length)
{
byte[] bytes = new byte[length];
for (int i = 0; i < length; i++)
{
bytes[i] = (byte)(value >> (i * 8));
}
return bytes;
}
```
其中,value是要转换的整数,length是结果字节数组的长度。该方法通过位移操作将整数的每个字节逐个提取出来,并存储到字节数组中。
相关问题
byte[] b3 = this.IntToByte((uint)r.RoadID, 2);
这段代码是将一个 uint 类型的 r.RoadID 转换成一个长度为 2 的 byte 数组。
函数 IntToByte 的实现可能如下:
```csharp
private byte[] IntToByte(uint value, int length)
{
byte[] result = new byte[length];
for (int i = 0; i < length; i++)
{
result[i] = (byte)(value >> (8 * i) & 0xff);
}
return result;
}
```
该函数首先创建了一个长度为 length 的 byte 数组 result。
然后,通过一个循环遍历数组,将 value 的每个字节存入 result 中。循环中,每次将 value 右移 8 * i 位,再与 0xff 进行按位与操作,就可以得到 value 的第 i 个字节。
最后,函数返回结果数组 result。
from libc.stdint cimport uint8_t
您好!您的第一个问题是关于 C 语言中导入 uint8_t 类型的问题。在 C 语言中,要导入 uint8_t 类型,可以使用 `#include <stdint.h>` 头文件,然后在代码中直接使用 uint8_t 类型,无需额外的导入操作。
如果您是在使用 Cython 编写 Python 扩展时,可以使用 `from libc.stdint cimport uint8_t` 来导入 uint8_t 类型。这将允许您在 Cython 代码中使用 uint8_t 类型,并与 C 代码进行交互。
请注意,Cython 是将 Python 代码转换为 C 代码的工具,因此在导入 C 数据类型时使用 `cimport` 是常见的做法。对于纯粹的 C 代码,可以直接使用 `#include <stdint.h>` 头文件来导入 uint8_t 类型。
希望能解答您的问题!如果您有任何其他问题,请随时提问。
相关推荐
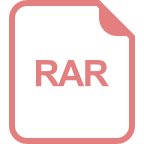
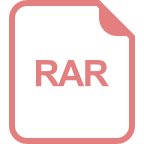












