Qt opengl assimp使用骨骼动画代码
时间: 2023-10-13 09:06:42 浏览: 229
以下是使用Qt、OpenGL和Assimp实现骨骼动画的示例代码:
首先,需要在项目中包含assimp库和Qt的OpenGL模块。可以使用以下命令将它们添加到.pro文件中:
```
LIBS += -lassimp
QT += opengl
```
然后,需要编写一个用于渲染模型的OpenGL窗口类。以下是一个简单的示例:
```cpp
#include <QOpenGLWidget>
#include <QOpenGLFunctions>
#include <assimp/Importer.hpp>
#include <assimp/scene.h>
#include <assimp/postprocess.h>
class OpenGLWidget : public QOpenGLWidget, protected QOpenGLFunctions
{
public:
OpenGLWidget(QWidget *parent = 0);
~OpenGLWidget();
protected:
void initializeGL();
void resizeGL(int w, int h);
void paintGL();
private:
Assimp::Importer m_importer;
const aiScene *m_scene;
};
```
在初始化函数中,需要加载模型并设置OpenGL状态:
```cpp
void OpenGLWidget::initializeGL()
{
initializeOpenGLFunctions();
// Load model
m_scene = m_importer.ReadFile("model.dae", aiProcess_Triangulate | aiProcess_GenSmoothNormals | aiProcess_FlipUVs | aiProcess_LimitBoneWeights);
// Set up OpenGL state
glEnable(GL_DEPTH_TEST);
glDepthFunc(GL_LESS);
glEnable(GL_BLEND);
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA);
}
```
在绘制函数中,需要遍历场景中的所有节点和网格,并计算每个节点的变换矩阵。以下是绘制函数的示例代码:
```cpp
void OpenGLWidget::paintGL()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
// Set up camera
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(45.0, (double)width() / (double)height(), 0.1, 100.0);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
gluLookAt(0.0, 0.0, 5.0, 0.0, 0.0, 0.0, 0.0, 1.0, 0.0);
// Draw meshes
for (unsigned int i = 0; i < m_scene->mNumMeshes; i++)
{
const aiMesh *mesh = m_scene->mMeshes[i];
// Set up vertex positions and normals
glEnableClientState(GL_VERTEX_ARRAY);
glVertexPointer(3, GL_FLOAT, 0, mesh->mVertices);
glEnableClientState(GL_NORMAL_ARRAY);
glNormalPointer(GL_FLOAT, 0, mesh->mNormals);
// Set up bone weights and indices
if (mesh->HasBones())
{
glEnableVertexAttribArray(4);
glVertexAttribPointer(4, 4, GL_FLOAT, GL_FALSE, sizeof(VertexBoneData), (const GLvoid*)offsetof(VertexBoneData, weights));
glEnableVertexAttribArray(5);
glVertexAttribPointer(5, 4, GL_FLOAT, GL_FALSE, sizeof(VertexBoneData), (const GLvoid*)offsetof(VertexBoneData, indices));
// Calculate bone matrices
std::vector<aiMatrix4x4> boneMatrices(mesh->mNumBones);
for (unsigned int j = 0; j < mesh->mNumBones; j++)
{
const aiBone *bone = mesh->mBones[j];
aiMatrix4x4 boneMatrix = bone->mOffsetMatrix;
const aiNode *node = m_scene->mRootNode->FindNode(bone->mName);
while (node && node != mesh->mBones[j]->mNode)
{
boneMatrix = node->mTransformation * boneMatrix;
node = node->mParent;
}
boneMatrices[j] = boneMatrix;
}
// Set up bone matrices
glUniformMatrix4fv(glGetUniformLocation(m_shaderProgram.programId(), "boneMatrices"), mesh->mNumBones, GL_FALSE, (const GLfloat*)&boneMatrices[0]);
}
// Draw triangles
glDrawElements(GL_TRIANGLES, mesh->mNumFaces * 3, GL_UNSIGNED_INT, mesh->mFaces[0].mIndices);
// Disable vertex and normal arrays
glDisableClientState(GL_VERTEX_ARRAY);
glDisableClientState(GL_NORMAL_ARRAY);
// Disable bone weights and indices
if (mesh->HasBones())
{
glDisableVertexAttribArray(4);
glDisableVertexAttribArray(5);
}
}
// Swap buffers
swapBuffers();
}
```
在这个示例中,假设模型使用了骨骼动画。因此,需要设置每个网格的顶点数据结构,其中包含每个顶点的骨骼权重和索引。以下是这个数据结构的示例代码:
```cpp
struct VertexBoneData
{
float weights[4];
unsigned int indices[4];
};
```
在顶点着色器中,需要将顶点位置和法线变换为相机空间,并使用骨骼权重和索引来计算每个顶点的最终位置。以下是顶点着色器的示例代码:
```glsl
#version 330
uniform mat4 projectionMatrix;
uniform mat4 modelViewMatrix;
uniform mat4 boneMatrices[100];
in vec3 vertexPosition;
in vec3 vertexNormal;
in vec4 vertexWeights;
in ivec4 vertexIndices;
out vec3 normal;
void main()
{
mat4 boneMatrix = boneMatrices[vertexIndices.x] * vertexWeights.x;
boneMatrix += boneMatrices[vertexIndices.y] * vertexWeights.y;
boneMatrix += boneMatrices[vertexIndices.z] * vertexWeights.z;
boneMatrix += boneMatrices[vertexIndices.w] * vertexWeights.w;
gl_Position = projectionMatrix * modelViewMatrix * boneMatrix * vec4(vertexPosition, 1.0);
normal = normalize(mat3(modelViewMatrix * boneMatrix) * vertexNormal);
}
```
在片段着色器中,可以使用法线和光照计算每个像素的颜色。以下是片段着色器的示例代码:
```glsl
#version 330
uniform vec3 lightPosition;
in vec3 normal;
out vec4 fragmentColor;
void main()
{
vec3 ambientColor = vec3(0.2, 0.2, 0.2);
vec3 diffuseColor = vec3(1.0, 1.0, 1.0);
vec3 specularColor = vec3(1.0, 1.0, 1.0);
float shininess = 50.0;
vec3 lightDirection = normalize(lightPosition - gl_FragCoord.xyz);
vec3 normalDirection = normalize(normal);
vec3 reflectionDirection = reflect(-lightDirection, normalDirection);
float diffuseFactor = max(dot(lightDirection, normalDirection), 0.0);
vec3 diffuseComponent = diffuseColor * diffuseFactor;
float specularFactor = pow(max(dot(reflectionDirection, normalize(-gl_FragCoord.xyz)), 0.0), shininess);
vec3 specularComponent = specularColor * specularFactor;
fragmentColor = vec4(ambientColor + diffuseComponent + specularComponent, 1.0);
}
```
最后,需要在Qt应用程序中创建OpenGL窗口并运行事件循环。以下是一个简单的示例:
```cpp
#include <QApplication>
#include "OpenGLWidget.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
OpenGLWidget w;
w.show();
return a.exec();
}
```
这就是使用Qt、OpenGL和Assimp实现骨骼动画的基本步骤和代码示例。
阅读全文
相关推荐
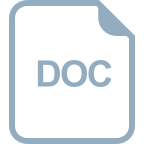
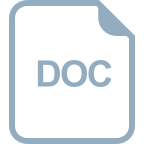
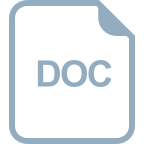


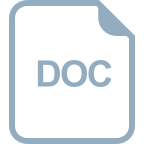
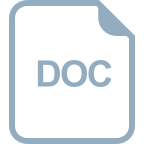
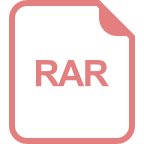
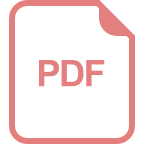
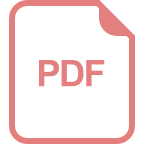
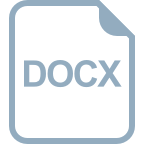
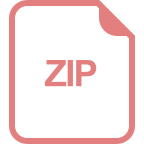
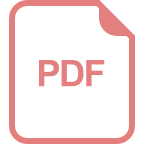
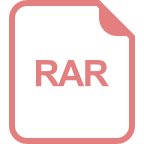
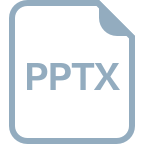
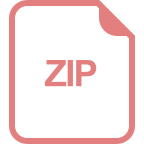
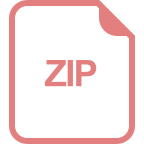