请用python代码写一个简单线性回归模型
时间: 2024-05-14 12:15:23 浏览: 101
好的,以下是一个简单的线性回归模型的Python代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 构造数据集
X = np.array([1, 2, 3, 4, 5])
y = np.array([1, 3, 2, 3, 5])
# 初始化参数
theta0 = 0
theta1 = 0
alpha = 0.01
iters = 1000
# 定义代价函数
def compute_cost(X, y, theta0, theta1):
m = len(y)
J = np.sum((theta0 + theta1*X - y)**2)/(2*m)
return J
# 执行批量梯度下降算法
def gradient_descent(X, y, theta0, theta1, alpha, iters):
m = len(y)
J_history = np.zeros(iters)
for i in range(iters):
theta0 = theta0 - alpha*np.sum(theta0 + theta1*X - y)/m
theta1 = theta1 - alpha*np.sum((theta0 + theta1*X - y)*X)/m
J_history[i] = compute_cost(X, y, theta0, theta1)
return theta0, theta1, J_history
# 执行梯度下降算法
theta0, theta1, J_history = gradient_descent(X, y, theta0, theta1, alpha, iters)
# 输出最终结果
print("theta0 = ", theta0)
print("theta1 = ", theta1)
# 绘制代价函数随迭代次数的变化曲线
plt.plot(range(iters), J_history)
plt.xlabel("Iterations")
plt.ylabel("Cost")
plt.show()
# 绘制数据点及最终拟合直线
plt.scatter(X, y)
plt.plot(X, theta0 + theta1*X, 'r-')
plt.xlabel("X")
plt.ylabel("y")
plt.show()
```
这个代码实现了一个简单的线性回归模型,并使用批量梯度下降算法对模型参数进行了优化。当然,这只是一个简单的示例,实际上线性回归模型的应用非常广泛,也有很多更加复杂的算法和技巧可以用来提高模型的性能。
阅读全文
相关推荐
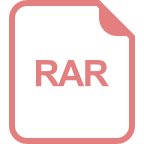
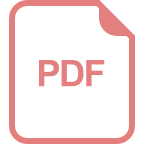













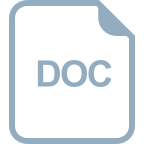
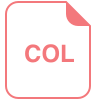
