写一个配置串口中断的配置,使用串口3中断发送一个字节,实现不断使用串口中断3发送10个字节的数据
时间: 2024-02-27 09:59:24 浏览: 91
好的,以下是一个配置串口中断的示例代码,实现了不断使用串口中断3发送10个字节的数据:
```c
#include <avr/io.h>
#include <avr/interrupt.h>
#define F_CPU 16000000UL
#define BAUD 9600
#define MYUBRR F_CPU/16/BAUD-1
volatile uint8_t tx_buffer[10] = {0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x0A};
volatile uint8_t tx_buffer_index = 0;
void USART_Init(unsigned int ubrr)
{
/* Set baud rate */
UBRR3H = (unsigned char)(ubrr >> 8);
UBRR3L = (unsigned char)ubrr;
/* Enable receiver and transmitter */
UCSR3B = (1 << RXEN3) | (1 << TXEN3) | (1 << TXCIE3);
/* Set frame format: 8data, 2stop bit */
UCSR3C = (1 << USBS3) | (3 << UCSZ30);
}
ISR(USART3_TX_vect)
{
/* Send next byte */
if (tx_buffer_index < 10) {
UDR3 = tx_buffer[tx_buffer_index++];
} else {
/* Disable UART TX interrupt */
UCSR3B &= ~(1 << TXCIE3);
}
}
int main(void)
{
USART_Init(MYUBRR);
/* Enable global interrupts */
sei();
/* Enable UART TX interrupt */
UCSR3B |= (1 << TXCIE3);
while (1);
}
```
在上述代码中,我们使用 USART3 来发送数据,`USART_Init` 函数用于初始化 USART3,`ISR(USART3_TX_vect)` 函数是 USART3 的中断处理函数。
在 `main` 函数中,我们首先调用 `USART_Init` 函数来初始化 USART3,然后打开全局中断使能,启用 UART 发送中断,并且进入一个死循环等待中断触发。
在 `ISR(USART3_TX_vect)` 函数中,我们先检查是否已经发送完了 10 个字节的数据,如果没有,则发送下一个字节;否则,禁用 UART 发送中断。
需要注意的是,这里使用了一个全局变量 `tx_buffer_index` 来记录当前发送到了第几个字节,这个变量在 `ISR(USART3_TX_vect)` 函数中被修改。
阅读全文
相关推荐
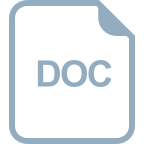
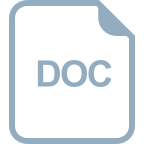
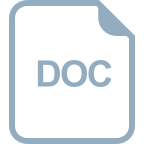

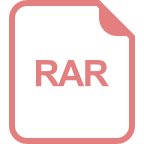
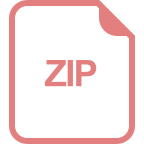












