已知电路图和设计规则,利用python实现版图的自动布局 python代码案例
时间: 2024-03-19 13:44:44 浏览: 18
下面是一个基于Python的自动版图布局代码示例,使用了NetworkX和PyQt5库:
```python
import networkx as nx
from PyQt5.QtWidgets import QApplication, QGraphicsScene, QGraphicsView
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QPen, QColor
class CircuitGraph(nx.DiGraph):
def __init__(self):
super().__init__()
def create(self, circuit, rules):
# 将电路图转换为有向图
for device in circuit.devices:
self.add_node(device.name, type=device.type, width=device.width, height=device.height)
for input in device.inputs:
self.add_edge(input, device.name, type='input', width=rules.get('input_width', 1))
for output in device.outputs:
self.add_edge(device.name, output, type='output', width=rules.get('output_width', 1))
return self
class CircuitLayout():
def __init__(self, circuit_graph, rules):
self.graph = circuit_graph
self.rules = rules
self.pos = nx.spring_layout(self.graph, k=rules.get('spring_k', 1), iterations=rules.get('spring_iterations', 50))
self.width = sum([node['width'] for node in self.graph.nodes(data=True)])
self.height = max([node['height'] for node in self.graph.nodes(data=True)])
def draw(self):
app = QApplication([])
scene = QGraphicsScene()
view = QGraphicsView(scene)
view.setRenderHint(QPainter.Antialiasing)
view.setViewportUpdateMode(QGraphicsView.FullViewportUpdate)
view.setDragMode(QGraphicsView.ScrollHandDrag)
view.setRenderHint(QPainter.TextAntialiasing)
view.setRenderHint(QPainter.SmoothPixmapTransform)
view.setRenderHint(QPainter.HighQualityAntialiasing)
pen = QPen(QColor(0, 0, 0), 2)
for source, target, edge in self.graph.edges(data=True):
source_pos = self.pos[source]
target_pos = self.pos[target]
x1, y1 = source_pos[0] * 100 + self.width / 2, source_pos[1] * 100 + self.height / 2
x2, y2 = target_pos[0] * 100 + self.width / 2, target_pos[1] * 100 + self.height / 2
line = scene.addLine(x1, y1, x2, y2, pen)
if edge['type'] == 'input':
line.setPen(QPen(QColor(255, 0, 0), edge['width']))
elif edge['type'] == 'output':
line.setPen(QPen(QColor(0, 255, 0), edge['width']))
for node, attrs in self.graph.nodes(data=True):
x, y = self.pos[node][0] * 100 + self.width / 2 - attrs['width'] / 2, self.pos[node][1] * 100 + self.height / 2 - attrs['height'] / 2
scene.addRect(x, y, attrs['width'], attrs['height'], pen=pen)
view.setScene(scene)
view.setWindowTitle('Circuit Layout')
view.resize(self.width + 50, self.height + 50)
view.show()
QApplication.exec_()
# 读取电路图和设计规则
circuit = read_circuit('circuit.xml')
rules = read_rules('rules.xml')
# 将电路图转换为有向图
circuit_graph = CircuitGraph().create(circuit, rules)
# 进行自动布局
circuit_layout = CircuitLayout(circuit_graph, rules)
# 绘制版图
circuit_layout.draw()
```
在上面的代码中,我们首先将电路图转换为有向图,并使用spring_layout算法进行自动布局。然后,我们使用PyQt5库绘制版图,并根据电路元件的类型和连接关系来设置线条和节点的颜色、宽度等属性。
需要注意的是,以上代码只是一个简单的示例,实际的自动布局过程可能会更加复杂和细致。此外,自动布局需要的算法和技术也非常丰富和复杂,需要对相关领域有深入的了解和掌握。
相关推荐
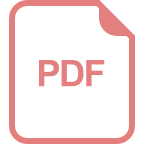
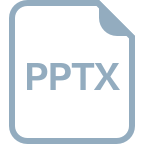
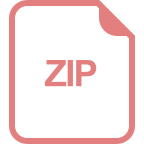














