CNN、LSTM以及attention机制进行股票预测的代码实现
时间: 2023-11-17 13:04:36 浏览: 114
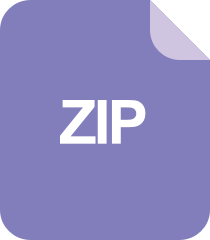
CNN-LSTM-Attention基于卷积-长短期记忆神经网络结合注意力机制的数据分类预测 Matlab语言 程序已调试好,无
股票预测是一个复杂的问题,需要考虑很多因素,包括股票市场的复杂性和不确定性。CNN、LSTM以及attention机制是当前比较流行的用于时间序列预测的技术,下面我来介绍一下如何使用这些技术进行股票预测的代码实现。
首先,我们需要准备股票数据。我们可以从Yahoo Finance上下载历史股票价格数据,并将其转换为时间序列。这里以苹果公司的股票为例,代码如下所示:
```python
import pandas as pd
import numpy as np
import yfinance as yf
data = yf.download('AAPL', start='2010-01-01', end='2021-06-01')
data = data.dropna()
prices = data['Close'].values
```
接下来,我们可以将股票价格数据分为训练集和测试集。我们可以使用训练集来训练模型,并使用测试集来评估模型的性能。这里我们将前80%的数据用于训练,后20%的数据用于测试。
```python
train_size = int(len(prices) * 0.8)
train_prices = prices[:train_size]
test_prices = prices[train_size:]
```
接下来,我们需要对数据进行预处理。我们可以使用归一化方法将数据缩放到[0,1]的范围内,以便它们可以被神经网络处理。这里我们使用Min-Max归一化方法。
```python
from sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
train_prices = scaler.fit_transform(train_prices.reshape(-1, 1))
test_prices = scaler.transform(test_prices.reshape(-1, 1))
```
接下来,我们可以开始构建模型。我们将使用CNN、LSTM以及attention机制来预测股票价格。
```python
from keras.layers import Input, Dense, Conv1D, MaxPooling1D, LSTM, Bidirectional, concatenate, Activation, Dot, Flatten
from keras.models import Model
from keras import backend as K
def attention_model(input_shape):
X_input = Input(input_shape)
conv1 = Conv1D(filters=32, kernel_size=5, activation='relu')(X_input)
pool1 = MaxPooling1D(pool_size=2)(conv1)
lstm1 = Bidirectional(LSTM(64, return_sequences=True))(pool1)
lstm2 = Bidirectional(LSTM(64))(lstm1)
attention_weights = Dense(1, activation='tanh')(lstm1)
attention_weights = Flatten()(attention_weights)
attention_weights = Activation('softmax')(attention_weights)
attention_weights = Dot(axes=1)([attention_weights, lstm1])
merged = concatenate([lstm2, attention_weights])
output = Dense(1)(merged)
model = Model(inputs=X_input, outputs=output)
return model
```
这里我们定义了一个attention模型,其输入为一维序列数据。在模型中,我们首先使用了一个卷积层和一个池化层来提取特征。然后,我们使用了一个双向LSTM层来学习时间序列的动态特征。接下来,我们使用了一个全连接层和一个softmax层来计算注意力权重,以便我们可以更好地关注重要的时间步。最后,我们将注意力权重和LSTM层的输出连接在一起,并使用一个全连接层来输出预测结果。
接下来,我们可以使用训练数据来训练模型。在训练过程中,我们将使用均方误差损失函数,并使用Adam优化器来更新模型参数。
```python
model = attention_model((train_prices.shape[0], 1))
model.compile(optimizer='adam', loss='mean_squared_error')
model.fit(train_prices.reshape(-1, 1, 1), train_prices, epochs=100, batch_size=64)
```
最后,我们可以使用测试数据来评估模型的性能。
```python
predictions = model.predict(test_prices.reshape(-1, 1, 1))
predictions = scaler.inverse_transform(predictions)
test_prices = scaler.inverse_transform(test_prices)
mse = np.mean((predictions - test_prices)**2)
print('MSE: ', mse)
```
这里我们将预测结果和测试数据反归一化,以便我们可以比较它们的实际值。然后,我们计算均方误差来评估模型的性能。
以上就是使用CNN、LSTM以及attention机制进行股票预测的代码实现。当然,这只是一个简单的示例,实际上股票预测是一个非常复杂的问题,需要考虑更多的因素。
阅读全文
相关推荐
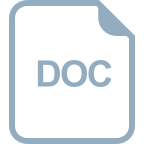
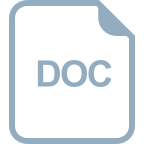



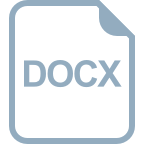
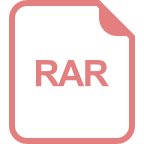
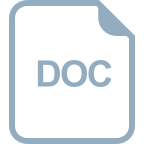
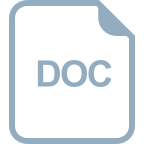







