凯撒密码c++加密及解密程序代码
时间: 2024-11-04 12:14:19 浏览: 73
凯撒密码是一种简单的替换式加密技术,它通过将明文中的每个字母按照字母表向前或向后移动固定的位置来进行加密。下面是一个简单的C++代码示例,演示了如何实现凯撒密码的加密和解密:
```cpp
#include <iostream>
#include <string>
std::string caesar_cipher_encrypt(const std::string& plaintext, int shift) {
std::string ciphertext;
for (char c : plaintext) {
if (isalpha(c)) { // 只处理字母字符
if (islower(c)) {
ciphertext += static_cast<char>((c + shift - 'a') % 26 + 'a');
} else {
ciphertext += static_cast<char>((c + shift - 'A') % 26 + 'A');
}
} else {
ciphertext += c; // 非字母字符保持不变
}
}
return ciphertext;
}
std::string caesar_cipher_decrypt(const std::string& ciphertext, int shift) {
return caesar_cipher_encrypt(ciphertext, -shift);
}
int main() {
std::string input("Hello, World!");
int shift = 3; // 示例加密位移
std::cout << "Original message: " << input << "\n";
std::string encrypted = caesar_cipher_encrypt(input, shift);
std::cout << "Encrypted message: " << encrypted << "\n";
std::string decrypted = caesar_cipher_decrypt(encrypted, shift);
std::cout << "Decrypted message: " << decrypted << "\n";
return 0;
}
```
在这个例子中,`caesar_cipher_encrypt`函数用于加密,`caesar_cipher_decrypt`函数用于解密。用户输入原始消息(如"Hello, World!"),并指定加密位移(例如3)。然后代码会输出加密后的消息以及解密的结果。
阅读全文
相关推荐





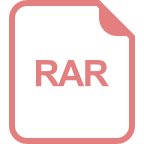

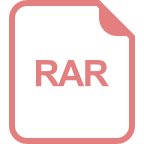










