ax.plot(new_name_lst, x_list,label='velocity_x')线条加粗
时间: 2024-01-21 19:19:00 浏览: 40
要将线条加粗,你可以在绘制时使用`linewidth`参数来指定线条宽度。例如:
```
ax.plot(new_name_lst, x_list, label='velocity_x', linewidth=2.5)
```
这里的`linewidth`参数值为2.5,你可以根据实际需要调整该值来改变线条宽度。
相关问题
fig, ax = plt.subplots() ax.plot(new_name_lst, x_list, label='velocity_x') ax.plot(new_name_lst, y_list, label='velocity_y') ax.plot(new_name_lst, list3, label='velocity') # leg = ax.legend(loc='upper left', fancybox=True, shadow=True) leg.get_frame().set_alpha(0.5) lines = [l for l in ax.lines] lined = dict() for legline, origline in zip(leg.get_lines(), lines): legline.set_picker(True) lined[legline] = origline def onpick(event): legline = event.artist origline = lined[legline] visible = not origline.get_visible() origline.set_visible(visible) if visible: legline.set_alpha(1.0) else: legline.set_alpha(0.2) fig.canvas.draw() fig.canvas.mpl_connect('pick_event', onpick) plt.show()这个代码加一个跟随鼠标的虚线
你可以尝试添加以下代码来实现跟随鼠标的虚线:
```python
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
# 假设你已经有了名为 new_name_lst、x_list、y_list 和 list3 的变量
ax.plot(new_name_lst, x_list, label='velocity_x')
ax.plot(new_name_lst, y_list, label='velocity_y')
ax.plot(new_name_lst, list3, label='velocity')
leg = ax.legend(loc='upper left', fancybox=True, shadow=True)
leg.get_frame().set_alpha(0.5)
lines = [l for l in ax.lines]
lined = dict()
for legline, origline in zip(leg.get_lines(), lines):
legline.set_picker(True)
lined[legline] = origline
def onpick(event):
legline = event.artist
origline = lined[legline]
visible = not origline.get_visible()
origline.set_visible(visible)
if visible:
legline.set_alpha(1.0)
else:
legline.set_alpha(0.2)
fig.canvas.draw()
# 添加跟随鼠标的虚线
if isinstance(event.artist, plt.Line2D):
xdata = event.artist.get_xdata()
ydata = event.artist.get_ydata()
x = event.mouseevent.xdata
y = event.mouseevent.ydata
idx = np.searchsorted(xdata, x)
if idx < len(xdata) and abs(x - xdata[idx]) > abs(x - xdata[idx-1]):
idx -= 1
if idx >= len(xdata) or idx == 0:
return
x = xdata[idx]
y = ydata[idx]
if not hasattr(ax, 'vline'):
ax.vline = ax.axvline(x=x, color='k', linestyle='--')
else:
ax.vline.set_xdata([x, x])
ax.vline.set_ydata([min(y, ax.get_ylim()[1]), max(y, ax.get_ylim()[0])])
fig.canvas.mpl_connect('pick_event', onpick)
plt.show()
```
这个代码会在图形中添加一个跟随鼠标的垂直虚线,当鼠标拾取到某个线条时,虚线会跟随鼠标移动到该线条上。
fig, ax1 = plt.subplots() ax1.plot(train_losses, label='Train Loss') ax1.plot(train_accs, label='Train Acc') ax1.set_ylim([0, 1]) ax1.yaxis.set_major_locator(y_major_locator) ax1.set_xlabel('Epoch') ax1.set_ylabel('Loss/Accuracy') ax1.legend() 使两条曲线线条有差别
要使两条曲线线条有差别,可以使用不同的颜色、线型或标记。以下是一些示例代码:
1. 使用不同的颜色:
```
fig, ax1 = plt.subplots()
ax1.plot(train_losses, label='Train Loss', color='red')
ax1.plot(train_accs, label='Train Acc', color='blue')
ax1.set_ylim([0, 1])
ax1.yaxis.set_major_locator(y_major_locator)
ax1.set_xlabel('Epoch')
ax1.set_ylabel('Loss/Accuracy')
ax1.legend()
```
2. 使用不同的线型:
```
fig, ax1 = plt.subplots()
ax1.plot(train_losses, label='Train Loss', linestyle='--')
ax1.plot(train_accs, label='Train Acc', linestyle=':')
ax1.set_ylim([0, 1])
ax1.yaxis.set_major_locator(y_major_locator)
ax1.set_xlabel('Epoch')
ax1.set_ylabel('Loss/Accuracy')
ax1.legend()
```
3. 使用不同的标记:
```
fig, ax1 = plt.subplots()
ax1.plot(train_losses, label='Train Loss', marker='o')
ax1.plot(train_accs, label='Train Acc', marker='s')
ax1.set_ylim([0, 1])
ax1.yaxis.set_major_locator(y_major_locator)
ax1.set_xlabel('Epoch')
ax1.set_ylabel('Loss/Accuracy')
ax1.legend()
```
你可以根据自己的需求选择其中一种或多种方法。
相关推荐
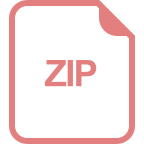













