如何在C++中正确地使用max函数来获取多个数的最大值?
时间: 2024-11-22 20:42:21 浏览: 0
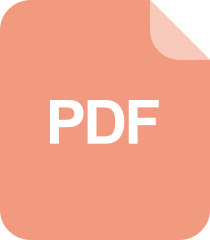
C++max函数的介绍及使用
在C++中,你可以使用`<algorithm>`库中的`std::max`函数来获取一组数值中的最大值。这个函数接受两个参数,并返回较大的那个。如果你想找出多个数中的最大值,可以采用循环或者模板元编程的方式。以下是基本步骤:
1. **使用循环**:
```cpp
#include <algorithm>
#include <vector>
int main() {
std::vector<int> numbers = {5, 10, 3, 8, 1};
int max_num = numbers[0]; // 先假设第一个元素是最大的
for (const auto& num : numbers) {
if (num > max_num) {
max_num = num;
}
}
std::cout << "最大值是: " << max_num << std::endl;
return 0;
}
```
2. **使用STL算法**:
如果你有不确定数量的输入,可以使用`std::accumulate`结合`std::partial_max_element`:
```cpp
#include <algorithm>
#include <numeric>
auto findMaxElement = [](const auto& a, const auto& b) -> decltype(auto) {
return a > b ? a : b;
};
int main() {
std::vector<int> numbers = {5, 10, 3, 8, 1};
int max_num = *std::partial_max_element(numbers.begin(), numbers.end(), findMaxElement);
std::cout << "最大值是: " << max_num << std::endl;
return 0;
}
```
这里`findMaxElement`是一个lambda表达式,它定义了比较规则。
阅读全文
相关推荐
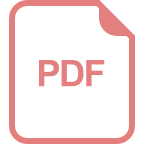














