C# 接收SQLserver变更通知
时间: 2024-11-30 09:16:03 浏览: 3
在C#中,接收SQL Server的变更通知通常涉及到数据库触发器(Database Triggers)和ADO.NET(ActiveX Data Objects for .NET)。你可以通过以下几个步骤实现:
1. **创建触发器**:
- 在SQL Server数据库中,编写一个或多个INSTEAD OF INSERT、UPDATE或DELETE触发器,这些触发器会在表数据发生变化时自动执行。
- 例如,你可以创建一个INSERT触发器,当新记录插入时发送一条消息到应用程序。
```sql
CREATE TRIGGER MyTrigger ON MyTable
AFTER INSERT
AS
BEGIN
SET NOCOUNT ON;
DECLARE @newId INT = scope_identity();
-- 发送消息给C#应用
EXEC msdb.dbo.sp_send_dbmail
@profile_name = 'MyMailProfile',
@recipients = 'appnotify@yourdomain.com',
@subject = 'New Record Added',
@body = N'Row inserted with ID: ' + CAST(@newId AS VARCHAR(10));
END;
```
2. **C#客户端监听**:
- 使用`SqlConnection`和`SqlCommand`创建一个连接,然后订阅数据库邮件服务(如SQL Mail)的消息接收。
- 可以利用SqlDependency或Entity Framework的ChangeTracker来检测数据库的变化。
```csharp
using System.Data.SqlClient;
using Microsoft.SqlServer.Management.Common;
// 创建依赖项对象
SqlDependency dependency = new SqlDependency("your_database", "your_table");
// 监听数据库更改
dependency.OnChange += Dependency_Changed;
public void Dependency_Changed(object sender, SqlNotificationEventArgs e)
{
if (e.ChangeType == SqlNotificationEventTypes.Insert)
{
// 处理新插入的数据
}
else if (e.ChangeType == SqlNotificationEventTypes.Update || e.ChangeType == SqlNotificationEventTypes.Delete)
{
// 处理更新或删除操作
}
}
// 注册并开始监听
dependency.Start();
```
阅读全文
相关推荐
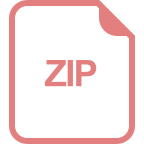
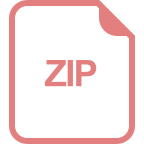



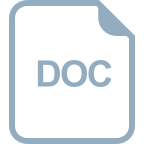
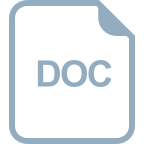
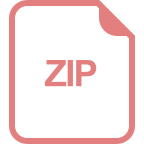
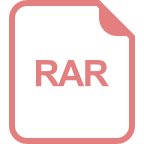
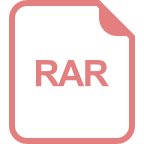
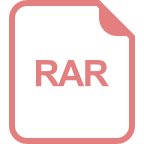
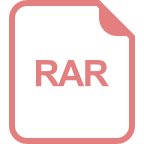
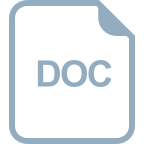
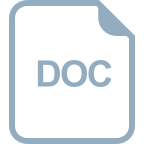
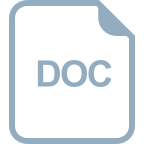

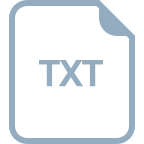
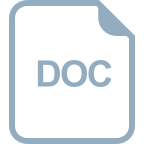