求任意阶方阵的逆矩阵c语言
时间: 2024-12-16 16:08:39 浏览: 26
求任意阶方阵的逆矩阵是一个常见的线性代数问题。在C语言中,我们可以通过高斯-约旦消元法来实现这一功能。以下是一个示例代码,演示如何求任意阶方阵的逆矩阵:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX 10
void getCofactor(float mat[MAX][MAX], float temp[MAX][MAX], int p, int q, int n);
float determinant(float mat[MAX][MAX], int n);
void adjoint(float mat[MAX][MAX], float adj[MAX][MAX]);
int inverse(float mat[MAX][MAX], float inverse[MAX][MAX], int n);
int main() {
float mat[MAX][MAX] = {
{5, -2, 2, 7},
{1, 0, 0, 3},
{-3, 1, 5, 0},
{3, -1, -9, 4}
};
float inv[MAX][MAX];
int n = 4;
if (inverse(mat, inv, n)) {
printf("The inverse is:\n");
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
printf("%f ", inv[i][j]);
}
printf("\n");
}
} else {
printf("The matrix is singular and cannot be inverted.\n");
}
return 0;
}
void getCofactor(float mat[MAX][MAX], float temp[MAX][MAX], int p, int q, int n) {
int i = 0, j = 0;
for (int row = 0; row < n; row++) {
for (int col = 0; col < n; col++) {
if (row != p && col != q) {
temp[i][j++] = mat[row][col];
if (j == n - 1) {
j = 0;
i++;
}
}
}
}
}
float determinant(float mat[MAX][MAX], int n) {
float D = 0;
if (n == 1) {
return mat[0][0];
}
float temp[MAX][MAX];
int sign = 1;
for (int f = 0; f < n; f++) {
getCofactor(mat, temp, 0, f, n);
D += sign * mat[0][f] * determinant(temp, n - 1);
sign = -sign;
}
return D;
}
void adjoint(float mat[MAX][MAX], float adj[MAX][MAX]) {
if (MAX == 1) {
adj[0][0] = 1;
return;
}
int sign = 1;
float temp[MAX][MAX];
for (int i = 0; i < MAX; i++) {
for (int j = 0; j < MAX; j++) {
getCofactor(mat, temp, i, j, MAX);
sign = ((i + j) % 2 == 0) ? 1 : -1;
adj[j][i] = (sign) * (determinant(temp, MAX - 1));
}
}
}
int inverse(float mat[MAX][MAX], float inverse[MAX][MAX], int n) {
float det = determinant(mat, n);
if (det == 0) {
return 0;
}
float adj[MAX][MAX];
adjoint(mat, adj);
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
inverse[i][j] = adj[i][j] / det;
}
}
return 1;
}
```
这个程序通过以下步骤求逆矩阵:
1. 计算矩阵的行列式。
2. 计算矩阵的伴随矩阵。
3. 用伴随矩阵除以行列式,得到逆矩阵。
请注意,这个程序假设输入矩阵是可逆的。如果矩阵不可逆,程序会输出错误信息。
阅读全文
相关推荐
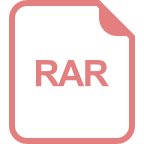
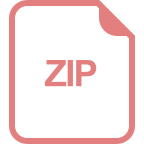
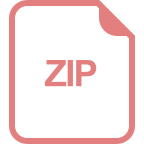
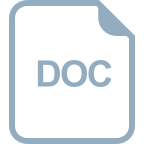
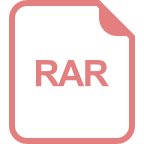
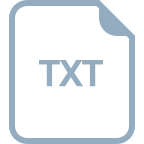
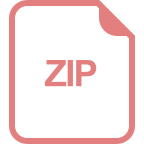
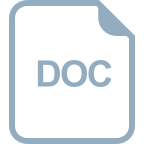
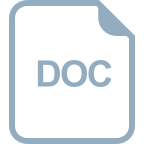
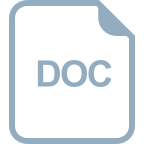
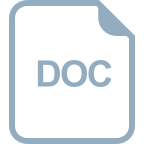
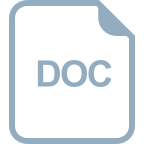
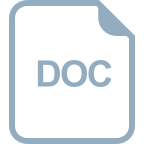




