帮我写一段代码,用qt实现控制鼠标点击的功能
时间: 2024-05-02 09:23:42 浏览: 107
好的,以下是一段用Qt实现控制鼠标点击功能的代码示例:
```cpp
#include <QCoreApplication>
#include <QCursor>
#include <QMouseEvent>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 模拟鼠标左键单击
QEvent clickEvent(QEvent::MouseButtonPress, QPoint(), Qt::LeftButton, Qt::LeftButton, Qt::NoModifier);
QCoreApplication::postEvent(QApplication::focusWidget(), &clickEvent);
QEvent releaseEvent(QEvent::MouseButtonRelease, QPoint(), Qt::LeftButton, Qt::LeftButton, Qt::NoModifier);
QCoreApplication::postEvent(QApplication::focusWidget(), &releaseEvent);
return a.exec();
}
```
这段代码会模拟鼠标左键单击事件,并发送到当前有焦点的窗口控件。你可以根据需要修改事件的参数,如鼠标按钮、坐标等。
相关问题
帮我写一段代码,用QT实现控制鼠标移动的功能
好的,以下是实现控制鼠标移动的代码示例:
```c++
#include <Windows.h>
#include <QtGui>
int main(int argc, char *argv[]) {
QApplication a(argc, argv);
QWidget w;
w.resize(300, 300);
w.move(0, 0);
w.show();
QCursor cursor;
// 控制鼠标移动至 x = 100,y = 100 的位置
cursor.setPos(100, 100);
// 让鼠标移动 50 个像素的距离
int dx = 50, dy = 50;
POINT p;
GetCursorPos(&p);
SetCursorPos(p.x + dx, p.y + dy);
return a.exec();
}
```
以上代码利用了 Windows 平台下的 API 函数,可以在 QT 中实现控制鼠标移动的功能。具体实现过程中,我们控制鼠标移动至指定位置的方式是通过 QCursor 类的 setPos() 方法。我们还可以让鼠标移动指定像素距离,利用 Windows API 函数 GetCursorPos() 和 SetCursorPos() 实现。
写一段在ros端进行鼠标点击控制发送串口信息的UI界面代码
以下是一个简单的ROS界面代码,可以通过鼠标点击来控制串口信息的发送:
```python
#!/usr/bin/env python
import rospy
import serial
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QVBoxLayout
from PyQt5.QtGui import QMouseEvent
# Serial Port Configuration
ser = serial.Serial('/dev/ttyACM0', 9600)
class ControlUI(QWidget):
def __init__(self):
super(ControlUI, self).__init__()
# UI Components
self.label = QLabel('Left click to send "Hello!" to serial port')
self.label.mousePressEvent = self.send_serial_data
# Layout
layout = QVBoxLayout()
layout.addWidget(self.label)
# Set layout
self.setLayout(layout)
def send_serial_data(self, event):
if isinstance(event, QMouseEvent) and event.button() == 1:
ser.write(b'Hello!')
if __name__ == '__main__':
# ROS Node Initialization
rospy.init_node('control_ui_node')
# PyQt5 Application Initialization
app = QApplication([])
control_ui = ControlUI()
control_ui.show()
# Start Application
app.exec_()
```
这个代码使用了 PyQt5 作为 UI 框架,当鼠标左键点击 Label 组件时,会向串口发送 "Hello!" 的数据。
阅读全文
相关推荐
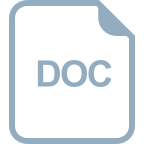
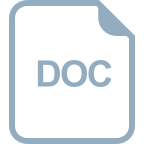
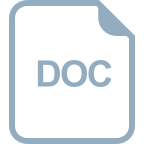
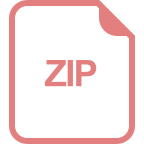
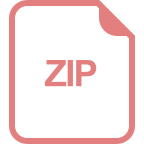
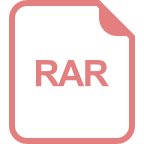
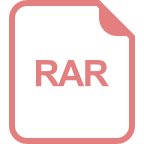
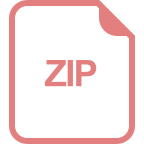
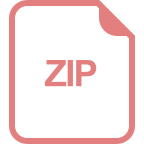
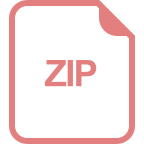
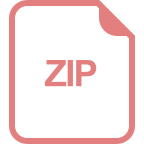
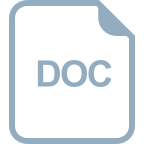
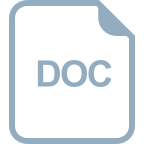
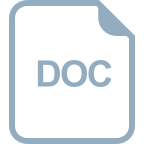
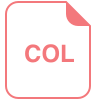

