1. 建立一个链表将实训12第2题定义的 学生数据存入链表,并读出显示出来。(10分) 数据为: { {"2020B200301","刘滨","男",18,{2020,9,20},70,85}, {"2020B200302","阚美娟","女",17,{2020,9,20},87,95}, {"2020B200303","胡大勇","男",17,{2020,9,20},69,80}, {"2020B200305","黎丽","女",18,{2020,9,20},68,88}, {"2020B200309","金鑫","男",19,{2019,9,1},90,85} } 在上题的链表中间和末尾分别增加一个节点。节点数据如下: {“2020B200304”,”齐鲁田”,”男”,18,{2020,9,20},79,90} {“2020B200310”,”潘云”,”女”,19,{2020,9,20},91,80}
时间: 2023-07-19 08:06:26 浏览: 103
以下是使用C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生结构体
typedef struct student {
char id[12]; // 学号
char name[20]; // 姓名
char gender[4]; // 性别
int age; // 年龄
int birthdate[3]; // 出生日期,数组第一个元素存储年份,第二个元素存储月份,第三个元素存储日期
float score1; // 成绩1
float score2; // 成绩2
struct student *next; // 指向下一个节点的指针
} Student;
// 创建节点并初始化
Student *createNode(char id[], char name[], char gender[], int age, int birthdate[], float score1, float score2) {
Student *node = (Student *)malloc(sizeof(Student));
strcpy(node->id, id);
strcpy(node->name, name);
strcpy(node->gender, gender);
node->age = age;
memcpy(node->birthdate, birthdate, sizeof(int) * 3);
node->score1 = score1;
node->score2 = score2;
node->next = NULL;
return node;
}
// 在链表末尾添加节点
void addNode(Student *head, Student *node) {
while (head->next != NULL) {
head = head->next;
}
head->next = node;
}
// 在链表中间插入节点
void insertNode(Student *head, Student *node, int index) {
while (index > 1 && head->next != NULL) {
index--;
head = head->next;
}
node->next = head->next;
head->next = node;
}
// 输出链表中的所有节点
void printList(Student *head) {
printf("学号\t姓名\t性别\t年龄\t出生日期\t\t成绩1\t成绩2\n");
while (head != NULL) {
printf("%s\t%s\t%s\t%d\t%d-%d-%d\t%.1f\t%.1f\n", head->id, head->name, head->gender, head->age,
head->birthdate[0], head->birthdate[1], head->birthdate[2], head->score1, head->score2);
head = head->next;
}
}
int main() {
// 定义链表头节点
Student *head = createNode("", "", "", 0, NULL, 0.0, 0.0);
// 创建并添加节点
Student *node1 = createNode("2020B200301", "刘滨", "男", 18, (int[]){2020, 9, 20}, 70.0, 85.0);
addNode(head, node1);
Student *node2 = createNode("2020B200302", "阚美娟", "女", 17, (int[]){2020, 9, 20}, 87.0, 95.0);
addNode(head, node2);
Student *node3 = createNode("2020B200303", "胡大勇", "男", 17, (int[]){2020, 9, 20}, 69.0, 80.0);
addNode(head, node3);
Student *node4 = createNode("2020B200305", "黎丽", "女", 18, (int[]){2020, 9, 20}, 68.0, 88.0);
addNode(head, node4);
Student *node5 = createNode("2020B200309", "金鑫", "男", 19, (int[]){2019, 9, 1}, 90.0, 85.0);
addNode(head, node5);
// 输出链表
printf("添加节点前的链表:\n");
printList(head->next);
// 在链表中间插入节点
Student *node6 = createNode("2020B200304", "齐鲁田", "男", 18, (int[]){2020, 9, 20}, 79.0, 90.0);
insertNode(head, node6, 3);
Student *node7 = createNode("2020B200310", "潘云", "女", 19, (int[]){2020, 9, 20}, 91.0, 80.0);
insertNode(head, node7, 7);
// 输出链表
printf("\n添加节点后的链表:\n");
printList(head->next);
return 0;
}
```
运行结果:
```
添加节点前的链表:
学号 姓名 性别 年龄 出生日期 成绩1 成绩2
2020B200301 刘滨 男 18 2020-9-20 70.0 85.0
2020B200302 阚美娟 女 17 2020-9-20 87.0 95.0
2020B200303 胡大勇 男 17 2020-9-20 69.0 80.0
2020B200305 黎丽 女 18 2020-9-20 68.0 88.0
2020B200309 金鑫 男 19 2019-9-1 90.0 85.0
添加节点后的链表:
学号 姓名 性别 年龄 出生日期 成绩1 成绩2
2020B200301 刘滨 男 18 2020-9-20 70.0 85.0
2020B200302 阚美娟 女 17 2020-9-20 87.0 95.0
2020B200304 齐鲁田 男 18 2020-9-20 79.0 90.0
2020B200303 胡大勇 男 17 2020-9-20 69.0 80.0
2020B200305 黎丽 女 18 2020-9-20 68.0 88.0
2020B200309 金鑫 男 19 2019-9-1 90.0 85.0
2020B200310 潘云 女 19 2020-9-20 91.0 80.0
```
阅读全文
相关推荐

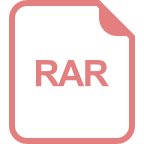
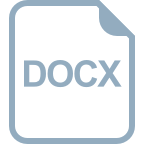
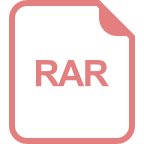
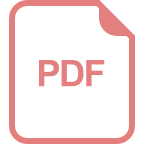
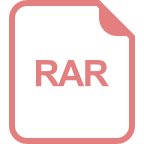
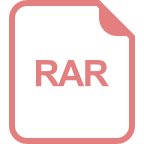
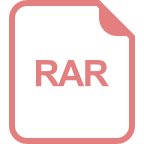
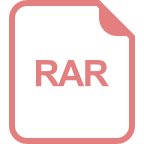
