笔记管理系统c语言
时间: 2023-07-03 18:21:22 浏览: 112
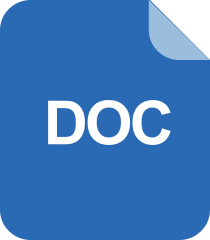
C语言笔记
好的,有关笔记管理系统的C语言实现,一般可以采用文件操作来实现数据的读写,以及结构体来存储笔记的相关信息。
以下是一个简单的笔记管理系统的C语言代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NOTES 100 // 最多笔记数量
#define MAX_TITLE_LENGTH 50 // 笔记标题最大长度
#define MAX_CONTENT_LENGTH 1000 // 笔记内容最大长度
// 笔记结构体
typedef struct {
char title[MAX_TITLE_LENGTH];
char content[MAX_CONTENT_LENGTH];
} Note;
// 读取笔记
int read_notes(Note *notes, int *count) {
FILE *fp;
fp = fopen("notes.txt", "r");
if (fp == NULL) {
return 0;
}
int i = 0;
while(!feof(fp)) {
fscanf(fp, "%[^\n]\n%[^\n]\n", notes[i].title, notes[i].content);
i++;
}
*count = i;
fclose(fp);
return 1;
}
// 保存笔记
int save_notes(Note *notes, int count) {
FILE *fp;
fp = fopen("notes.txt", "w");
if (fp == NULL) {
return 0;
}
for (int i = 0; i < count; i++) {
fprintf(fp, "%s\n%s\n", notes[i].title, notes[i].content);
}
fclose(fp);
return 1;
}
// 添加笔记
void add_note() {
Note new_note;
printf("请输入笔记标题:\n");
scanf("%s", new_note.title);
printf("请输入笔记内容:\n");
scanf("%s", new_note.content);
Note notes[MAX_NOTES];
int count;
if (read_notes(notes, &count)) {
notes[count] = new_note;
count++;
if (save_notes(notes, count)) {
printf("笔记添加成功!\n");
} else {
printf("笔记添加失败!\n");
}
} else {
printf("读取笔记失败!\n");
}
}
// 查看笔记
void view_notes() {
Note notes[MAX_NOTES];
int count;
if (read_notes(notes, &count)) {
printf("共有%d条笔记:\n", count);
for (int i = 0; i < count; i++) {
printf("标题:%s\n", notes[i].title);
printf("内容:%s\n", notes[i].content);
printf("\n");
}
} else {
printf("读取笔记失败!\n");
}
}
// 主函数
int main() {
int choice;
do {
printf("请选择操作:\n");
printf("1. 添加笔记\n");
printf("2. 查看笔记\n");
printf("0. 退出\n");
scanf("%d", &choice);
switch(choice) {
case 1:
add_note();
break;
case 2:
view_notes();
break;
case 0:
printf("退出笔记管理系统!\n");
break;
default:
printf("无效的选择!\n");
}
} while(choice != 0);
return 0;
}
```
以上是一个简单的笔记管理系统的C语言实现示例。当然,实际开发中需要根据实际需求进行适当的修改和完善。
阅读全文
相关推荐
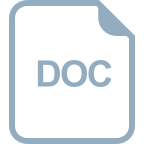
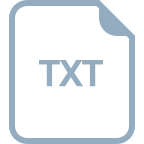
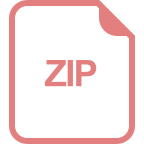
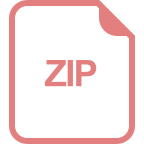
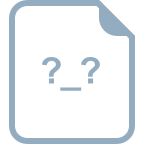
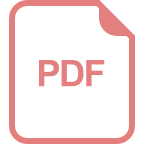
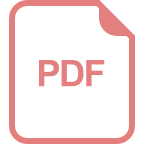
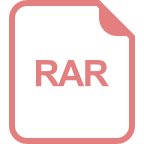
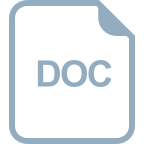
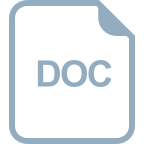
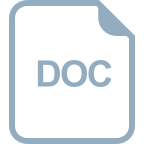



