如何在C++中正确地声明节点(Nodes)?
时间: 2024-12-13 13:24:45 浏览: 3
在C++中,声明一个节点通常涉及到定义一个结构体(struct)或类(class),用于表示数据和连接到其他节点的关系。下面是一个简单的例子,我们创建一个名为`Node`的类,它有一个值域`data`和一个指向下一个节点的指针`next`:
```cpp
// 如果是链表节点
class Node {
public:
// 数据成员
int data;
// 指向下一个节点的指针(如果是单链表)
Node* next;
// 构造函数用于初始化节点
Node(int value = 0) : data(value), next(nullptr) {}
};
// 如果是树形结构中的节点,可以添加更多的属性和指针
class TreeNode {
public:
int data;
TreeNode* left; // 左孩子指针
TreeNode* right; // 右孩子指针
TreeNode(int value = 0) : data(value), left(nullptr), right(nullptr) {}
};
```
相关问题
如何用C++实现二叉树的数据结构,包括计算叶子节点的数量、每个节点的深度以及总节点数?
在C++中,我们可以使用结构体或者类来实现二叉树的数据结构。这里我们首先定义一个简单的二叉树节点类,包含左孩子指针、右孩子指针和值:
```cpp
class TreeNode {
public:
int val;
TreeNode* left;
TreeNode* right;
// 构造函数和默认构造函数
TreeNode(int val = 0) : val(val), left(nullptr), right(nullptr) {}
};
```
接下来,我们可以创建一个二叉树类,包含计算叶子节点、节点深度和总数的方法:
```cpp
class BinaryTree {
private:
TreeNode* root;
public:
BinaryTree(TreeNode* root = nullptr) : root(root) {}
// 计算叶子节点数量
int countLeaves() const {
return countLeavesHelper(root);
}
private:
int countLeavesHelper(TreeNode* node) const {
if (!node) return 0; // 空节点无叶子
if (!node->left && !node->right) return 1; // 非空叶节点计数
else return countLeavesHelper(node->left) + countLeavesHelper(node->right);
}
// 计算节点深度 (假设根节点深度为1)
int depth(TreeNode* node) const {
if (!node) return 0;
return 1 + std::max(depth(node->left), depth(node->right));
}
// 总节点数
int totalNodes() const {
return totalNodesHelper(root);
}
private:
int totalNodesHelper(TreeNode* node) const {
if (!node) return 0;
return 1 + totalNodesHelper(node->left) + totalNodesHelper(node->right);
}
};
```
现在你可以创建一个`BinaryTree`实例并调用上述方法来获取你需要的信息。例如:
```cpp
int main() {
// 创建二叉树...
BinaryTree tree;
int leafCount = tree.countLeaves();
int maxDepth = tree.depth(tree.root);
int totalNodes = tree.totalNodes();
std::cout << "Leaf nodes: " << leafCount << std::endl;
std::cout << "Max depth: " << maxDepth << std::endl;
std::cout << "Total nodes: " << totalNodes << std::endl;
return 0;
}
```
c++编写形成节点导纳矩阵
在C++中,节点导纳矩阵是电力系统分析中的一个关键概念,用于表示电路中各个节点之间的电流关联。这个矩阵通常是一个方阵,其中的元素n[i][j]代表从节点i到节点j的导纳值(可能是电阻、电感或电容)。如果节点i和j之间没有直接连接,则对应元素为0。
以下是构建节点导纳矩阵的一个基本步骤:
1. **定义数据结构**:首先,创建一个适当的数据结构(如邻接表或邻接矩阵),存储网络的连接信息,包括每个节点的邻居以及相应的导纳值。
```cpp
struct Node {
int index;
std::vector<Node*> neighbors; // 存储相邻节点及其导纳
double conductance; // 导纳值
};
```
2. **初始化矩阵**:创建一个二维动态数组或矩阵,其大小等于节点数。对于每一对相连的节点,将它们的导纳值加到对应的矩阵元素上,并处理对角线(自环)的导纳。
```cpp
std::vector<std::vector<double>> nodeMatrix(Nodes.size(), std::vector<double>(Nodes.size(), 0.0));
for (const auto& node : Nodes) {
for (auto neighbor : node.neighbors) {
nodeMatrix[node.index][neighbor-node.conductance; // 负号是因为导纳矩阵是对称的
nodeMatrix[neighbor->index][node.index] = -node.conductance;
}
if (node != neighbor) { // 避免自环的贡献
nodeMatrix[node.index][node.index] -= node.conductance;
}
}
```
3. **调整矩阵**:在一些情况下,可能需要将矩阵转换为阻抗矩阵(R + jX),这涉及到取实部或虚部。
注意:上述代码只是一个简化的示例,实际应用中可能还需要考虑其他因素,比如分支的方向(有向图)或非对称元件的情况。
阅读全文
相关推荐
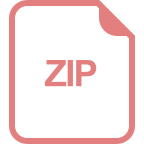
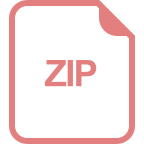
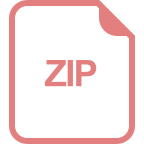
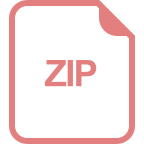
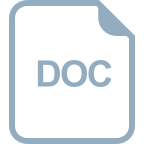











