C语言完整代码实现二叉树的递归先序中序后序遍历以及非递归层次遍历
时间: 2023-08-30 11:09:12 浏览: 47
```
#include<stdio.h>
#include<stdlib.h>
//定义二叉树结构体
struct TreeNode {
int data;
struct TreeNode *left;
struct TreeNode *right;
};
//创建节点函数
struct TreeNode *createNode(int data) {
struct TreeNode *newNode = (struct TreeNode *)malloc(sizeof(struct TreeNode));
newNode->data = data;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
//插入节点函数
struct TreeNode *insert(struct TreeNode *root, int data) {
if (root == NULL) {
return createNode(data);
} else if (data <= root->data) {
root->left = insert(root->left, data);
} else {
root->right = insert(root->right, data);
}
return root;
}
//递归先序遍历函数
void preOrder(struct TreeNode *root) {
if (root == NULL) {
return;
}
printf("%d ", root->data);
preOrder(root->left);
preOrder(root->right);
}
//递归中序遍历函数
void inOrder(struct TreeNode *root) {
if (root == NULL) {
return;
}
inOrder(root->left);
printf("%d ", root->data);
inOrder(root->right);
}
//递归后序遍历函数
void postOrder(struct TreeNode *root) {
if (root == NULL) {
return;
}
postOrder(root->left);
postOrder(root->right);
printf("%d ", root->data);
}
//非递归层次遍历函数
void levelOrder(struct TreeNode *root) {
if (root == NULL) {
return;
}
struct TreeNode *queue[100];
int front = 0;
int rear = 0;
queue[rear] = root;
rear++;
while (front <= rear) {
struct TreeNode *current = queue[front];
front++;
printf("%d ", current->data);
if (current->left != NULL) {
queue[rear] = current->left;
rear++;
}
if (current->right != NULL) {
queue[rear] = current->right;
rear++;
}
}
}
int main() {
struct TreeNode *root = NULL;
root = insert(root, 5);
root = insert(root, 3);
root = insert(root, 7);
root = insert(root, 2);
root = insert(root, 4);
root = insert(root, 6);
root = insert(root, 8);
printf("递归先序遍历:");
preOrder(root);
printf("\n");
printf("递归中序遍历:");
inOrder(root);
printf("\n");
printf("递归后序遍历:");
postOrder(root);
printf("\n");
printf("非递归层次遍历:");
levelOrder(root);
printf("\n");
return 0;
}
```
相关推荐
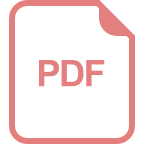
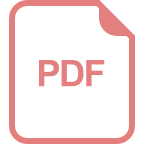
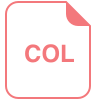













